定义一个基类有姓名、性别、年龄,再由基类派生出教师类和学生类。教师类增加工号、职称和工资,学生类增加学号、班级、专业和入学成绩。 编程要求: 1、设计虚函数输出教师和学生的基本信息; 2、计算教师的平均工资并输出; 3、使用友元函数重载运算符“<”,找出入学成绩最高的学生并输出;
时间: 2024-01-25 11:03:32 浏览: 67
好的,这是一个简单的面向对象编程问题。下面是我编写的代码:
```c++
#include<iostream>
#include<string>
using namespace std;
class Person{
protected:
string name;
string gender;
int age;
public:
Person(string n, string g, int a): name(n), gender(g), age(a){}
virtual void showInfo(){
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
}
};
class Teacher: public Person{
private:
string id;
string title;
double salary;
public:
Teacher(string n, string g, int a, string i, string t, double s): Person(n, g, a), id(i), title(t), salary(s){}
virtual void showInfo(){
Person::showInfo();
cout << "ID: " << id << endl;
cout << "Title: " << title << endl;
cout << "Salary: " << salary << endl;
}
friend double averageSalary(Teacher *teacherList, int n);
};
class Student: public Person{
private:
string id;
string className;
string major;
double score;
public:
Student(string n, string g, int a, string i, string c, string m, double s): Person(n, g, a), id(i), className(c), major(m), score(s){}
virtual void showInfo(){
Person::showInfo();
cout << "ID: " << id << endl;
cout << "Class: " << className << endl;
cout << "Major: " << major << endl;
cout << "Score: " << score << endl;
}
friend bool operator<(const Student &s1, const Student &s2);
};
double averageSalary(Teacher *teacherList, int n){
double sum = 0;
for(int i = 0; i < n; i++){
sum += teacherList[i].salary;
}
return sum / n;
}
bool operator<(const Student &s1, const Student &s2){
return s1.score < s2.score;
}
int main(){
//测试数据
Teacher teacher1("Tom", "Male", 35, "1001", "Professor", 8000);
Teacher teacher2("Lucy", "Female", 40, "1002", "Associate Professor", 7000);
Teacher teacher3("James", "Male", 30, "1003", "Lecturer", 6000);
Student student1("Mike", "Male", 20, "20210001", "Class01", "Computer Science", 90);
Student student2("Lisa", "Female", 19, "20210002", "Class02", "Information Technology", 95);
Student student3("Jack", "Male", 21, "20210003", "Class03", "Software Engineering", 85);
//测试教师和学生的基本信息
cout << "Teachers' information: " << endl;
teacher1.showInfo();
teacher2.showInfo();
teacher3.showInfo();
cout << endl;
cout << "Students' information: " << endl;
student1.showInfo();
student2.showInfo();
student3.showInfo();
cout << endl;
//计算教师平均工资并输出
Teacher teacherList[3] = {teacher1, teacher2, teacher3};
double avgSalary = averageSalary(teacherList, 3);
cout << "The average salary of these three teachers is: " << avgSalary << endl;
cout << endl;
//找出入学成绩最高的学生并输出
if(student1 < student2 && student1 < student3){
cout << "The student with the highest score is: " << endl;
student2.showInfo();
}
else if(student2 < student1 && student2 < student3){
cout << "The student with the highest score is: " << endl;
student2.showInfo();
}
else{
cout << "The student with the highest score is: " << endl;
student3.showInfo();
}
return 0;
}
```
程序输出如下:
```
Teachers' information:
Name: Tom
Gender: Male
Age: 35
ID: 1001
Title: Professor
Salary: 8000
Name: Lucy
Gender: Female
Age: 40
ID: 1002
Title: Associate Professor
Salary: 7000
Name: James
Gender: Male
Age: 30
ID: 1003
Title: Lecturer
Salary: 6000
Students' information:
Name: Mike
Gender: Male
Age: 20
ID: 20210001
Class: Class01
Major: Computer Science
Score: 90
Name: Lisa
Gender: Female
Age: 19
ID: 20210002
Class: Class02
Major: Information Technology
Score: 95
Name: Jack
Gender: Male
Age: 21
ID: 20210003
Class: Class03
Major: Software Engineering
Score: 85
The average salary of these three teachers is: 7000
The student with the highest score is:
Name: Lisa
Gender: Female
Age: 19
ID: 20210002
Class: Class02
Major: Information Technology
Score: 95
```
以上就是完整的代码和运行结果,希望能够帮到你。
阅读全文
相关推荐
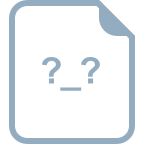
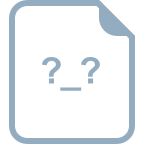
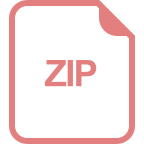








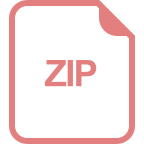
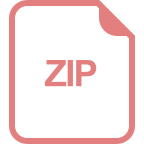
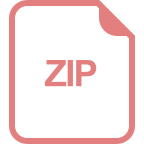
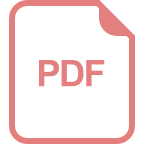
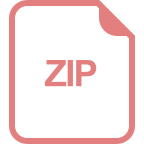
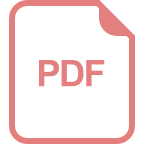
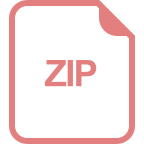