jdk17 自带的加密算法 实现
时间: 2023-09-02 12:11:10 浏览: 93
JDK 17 自带了多种加密算法,可以通过Java标准库中的 `javax.crypto` 包进行实现。下面是一个使用 JDK 17 自带的 AES 算法进行加密和解密的实现代码:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
public class AESEncryptionExample {
private static final String ALGORITHM = "AES";
private static final int KEY_SIZE = 128;
public static byte[] encrypt(String plainText, String key) throws Exception {
byte[] keyBytes = key.getBytes(StandardCharsets.UTF_8);
byte[] plainTextBytes = plainText.getBytes(StandardCharsets.UTF_8);
// Create a secret key from the given key bytes
SecretKeySpec keySpec = new SecretKeySpec(keyBytes, ALGORITHM);
// Initialize the cipher with the secret key and encrypt mode
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
// Encrypt the plain text
byte[] encryptedTextBytes = cipher.doFinal(plainTextBytes);
return encryptedTextBytes;
}
public static String decrypt(byte[] encryptedText, String key) throws Exception {
byte[] keyBytes = key.getBytes(StandardCharsets.UTF_8);
// Create a secret key from the given key bytes
SecretKeySpec keySpec = new SecretKeySpec(keyBytes, ALGORITHM);
// Initialize the cipher with the secret key and decrypt mode
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, keySpec);
// Decrypt the encrypted text
byte[] decryptedTextBytes = cipher.doFinal(encryptedText);
return new String(decryptedTextBytes, StandardCharsets.UTF_8);
}
public static String generateKey() throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);
SecureRandom secureRandom = new SecureRandom();
keyGenerator.init(KEY_SIZE, secureRandom);
SecretKey secretKey = keyGenerator.generateKey();
byte[] keyBytes = secretKey.getEncoded();
return new String(keyBytes, StandardCharsets.UTF_8);
}
}
```
这个实现包括了 AES 加密和解密的方法,使用时需要提供明文、密钥,或者密文和密钥。`generateKey` 方法可以生成一个随机的 AES 密钥,可以用于加密和解密。在实际使用中,需要注意密钥的生成和管理,以及使用恰当的加密算法和模式。
相关推荐
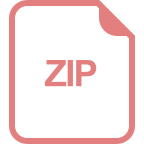
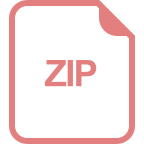
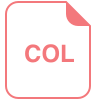
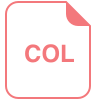
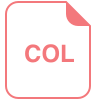











