用Python创建一个单循环链表
时间: 2023-11-16 15:04:37 浏览: 80
以下是使用Python创建单循环链表的示例代码:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class CircularLinkedList:
def __init__(self):
self.head = None
def add_node(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
new_node.next = self.head
else:
curr_node = self.head
while curr_node.next != self.head:
curr_node = curr_node.next
curr_node.next = new_node
new_node.next = self.head
def display(self):
curr_node = self.head
while curr_node.next != self.head:
print(curr_node.data, end=" -> ")
curr_node = curr_node.next
print(curr_node.data)
# Create a circular linked list
cll = CircularLinkedList()
cll.add_node(10)
cll.add_node(20)
cll.add_node(30)
cll.add_node(40)
# Display the circular linked list
cll.display()
```
输出:
```
10 -> 20 -> 30 -> 40
```
阅读全文
相关推荐
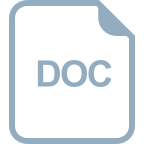
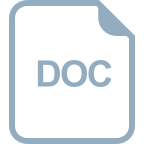
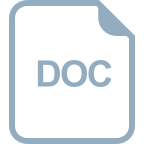

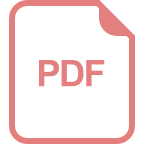
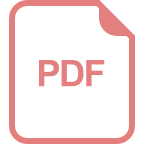
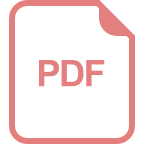










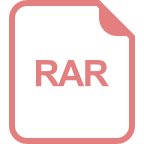
