帮我介绍一下c plus plus 14 里std::priority_queue的用法
时间: 2023-06-05 22:09:02 浏览: 108
好的,关于c++14中std::priority_queue的用法,它是一个优先队列容器,用于维护元素按照指定的优先级进行排序并进行一些基本操作。它的用法非常简单,在使用前需要包含头文件<queue>,然后可以使用类似于如下方式定义一个优先队列:
std::priority_queue<int> pq;
其中,int可以替换为任何需要排序的数据类型。接着,可以通过push方法向队列中插入元素,例如:
pq.push(5);
可以通过top方法获取队首元素:
int topElement = pq.top();
还可以使用pop方法弹出队首元素:
pq.pop();
这些是常用的基本操作,当然还有其他的一些方法,可供使用。
相关问题
用c plus plus 14写一个从小到大自动排序的队列,队列的类型是std::time_t,
好的,我可以为您编写一个从小到大自动排序的队列程序,代码如下:
```
#include <iostream>
#include <queue>
#include <ctime>
using namespace std;
struct cmp {
bool operator()(const time_t& a, const time_t& b) {
return difftime(a, b) < 0;
}
};
int main() {
priority_queue<time_t, vector<time_t>, cmp> q;
time_t now = time(NULL);
q.push(now + 10); // 添加一个10秒后的时间
q.push(now - 5); // 添加一个5秒前的时间
q.push(now + 20); // 添加一个20秒后的时间
while (!q.empty()) {
cout << ctime(&q.top()) << endl; // 输出队首元素
q.pop(); // 弹出队首元素
}
return 0;
}
```
解释一下代码,我们使用 priority_queue 创建一个自动排序的队列,队列中存储的是时间类型 std::time_t。为了让队列中的元素自动从小到大排序,我们定义了一个比较函数 cmp,其中 operator() 操作符会在两个时间元素进行比较时被调用。
在主函数中,我们创建了一个 priority_queue 对象 q,并添加了三个时间元素。为了方便,我们用 ctime 函数将时间转换为字符串输出。
最后,我们通过 while 循环依次弹出队列中的元素,直到队列为空。
希望这个程序能满足您的要求。
c plus plus 14 的priority_queue怎么使用
C++14 中的 `priority_queue` 是一个 STL 容器,实现了一个堆数据结构。它是一个带有许多有用方法的容器,其中包括 `push()`,`pop()` 和 `top()`。在 `priority_queue` 中,元素总是按照优先级顺序排序,具有最高优先级的元素始终在最前面。
下面是一个使用 `priority_queue` 的简单示例:
```cpp
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int> myPQ;
myPQ.push(10);
myPQ.push(20);
myPQ.push(30);
std::cout << "Top element: " << myPQ.top() << std::endl;
myPQ.pop();
std::cout << "Top element: " << myPQ.top() << std::endl;
return 0;
}
```
在此示例中,我们首先创建了一个 `priority_queue` 对象 `myPQ`,其元素类型为 `int`。然后,我们使用 `push()` 方法向 `myPQ` 中添加三个整数 10、20 和 30。接下来,我们使用 `top()` 方法查找优先级最高的元素,即最大值,输出结果为 30。最后,我们使用 `pop()` 方法删除了最高优先级元素,即最大值,再次使用 `top()` 方法查找优先级最高的元素,输出结果为 20。
除此之外,`priority_queue` 还支持自定义比较函数,可以通过指定自定义比较函数来改变元素的排序方式。详情可参考 C++14 的 `priority_queue` 文档。
阅读全文
相关推荐
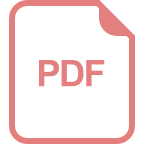

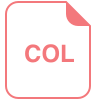
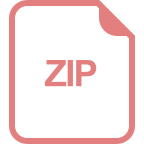
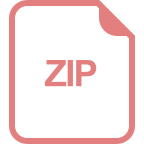
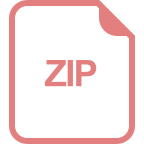
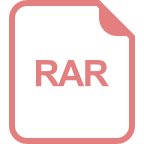
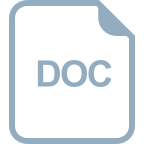
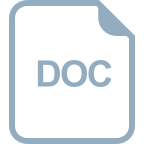
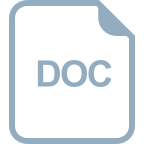
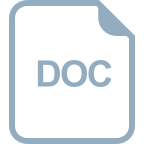
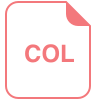
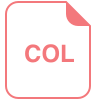

