用 C plus plus 写分支限界算法
时间: 2024-01-02 21:05:07 浏览: 12
以下是用 C++ 写的基本分支限界算法:
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
const int MAXN = 100; // 最大节点数
const int INF = 0x3f3f3f3f; // 无穷大
int n; // 节点数
int w[MAXN][MAXN]; // 图的邻接矩阵
int best; // 当前最优解
bool vis[MAXN]; // 标记节点是否被访问过
struct Node {
int u; // 当前节点
int w; // 当前节点的权值
int cost; // 当前路径的总权值
bool operator<(const Node& other) const {
return cost > other.cost; // 按路径总权值从小到大排序
}
};
int branchBound() {
priority_queue<Node> q;
Node node = {0, 0, 0}; // 初始节点
q.push(node);
while (!q.empty()) {
node = q.top();
q.pop();
if (node.cost >= best) { // 如果当前路径的总权值已经大于当前最优解,则剪枝
continue;
}
if (node.u == n - 1) { // 已到达终点
best = node.cost;
continue;
}
for (int i = 0; i < n; i++) {
if (w[node.u][i] != INF && !vis[i]) { // 如果当前节点可以到达节点 i,并且节点 i 未被访问过
vis[i] = true;
Node nextNode = {i, w[node.u][i], node.cost + w[node.u][i]};
q.push(nextNode);
vis[i] = false;
}
}
}
return best;
}
int main() {
cin >> n;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> w[i][j];
if (w[i][j] == -1) {
w[i][j] = INF; // 将边权为 -1 的边变成无穷大
}
}
}
best = INF;
vis[0] = true;
cout << branchBound() << endl;
return 0;
}
相关推荐
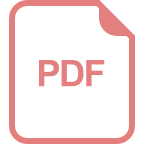
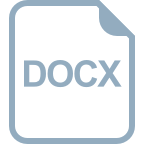














