用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,使用opencv, 两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us5’,自然图像的文件路径是‘D:\zzz\na4’,两种图像都有84张,图像的名称都是1到84的顺序数,两种图像的形状大小相同,需要对比两种图像的特征必须有颜色,纹理,形态学特征,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-16 20:06:13 浏览: 32
为了实现这个课题,我们需要使用以下模块:
- OpenCV:用于图像处理和分析
- NumPy:用于数值计算和数组处理
- Matplotlib:用于可视化
首先,我们需要导入这些模块:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
```
接下来,我们需要定义函数来加载图像并提取它们的特征。我们将使用颜色直方图、灰度共生矩阵和形态学特征。
```python
def load_images(path):
images = []
for i in range(1, 85):
filename = f"{path}/{i}.jpg"
img = cv2.imread(filename)
images.append(img)
return images
def get_color_histogram(img):
hist = cv2.calcHist([img], [0, 1, 2], None, [8, 8, 8], [0, 256, 0, 256, 0, 256])
hist = cv2.normalize(hist, hist).flatten()
return hist
def get_gray_cooccurrence(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cooccurrence = np.zeros((256, 256))
for i in range(gray.shape[0]):
for j in range(gray.shape[1] - 1):
cooccurrence[gray[i, j], gray[i, j+1]] += 1
cooccurrence = cv2.normalize(cooccurrence, cooccurrence).flatten()
return cooccurrence
def get_morphological_features(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
kernel = np.ones((3, 3), np.uint8)
opening = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel, iterations=2)
closing = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel, iterations=2)
gradient = cv2.morphologyEx(thresh, cv2.MORPH_GRADIENT, kernel)
features = [np.mean(opening), np.mean(closing), np.mean(gradient), np.std(opening), np.std(closing), np.std(gradient)]
return features
```
现在,我们可以使用这些函数来加载图像并提取它们的特征:
```python
us_images = load_images('D:/zzz/us5')
na_images = load_images('D:/zzz/na4')
us_color_histograms = [get_color_histogram(img) for img in us_images]
na_color_histograms = [get_color_histogram(img) for img in na_images]
us_gray_cooc = [get_gray_cooccurrence(img) for img in us_images]
na_gray_cooc = [get_gray_cooccurrence(img) for img in na_images]
us_morphological = [get_morphological_features(img) for img in us_images]
na_morphological = [get_morphological_features(img) for img in na_images]
```
现在,我们可以使用这些特征来进行两种图像之间的差异性分析。我们将使用Kolmogorov-Smirnov测试来比较颜色直方图和灰度共生矩阵特征的分布,使用t-test来比较形态学特征的均值和标准差。
```python
def compare_features(f1, f2, test):
scores = []
for i in range(len(f1)):
score = test(f1[i], f2[i])[1]
scores.append(score)
return scores
def plot_distribution_scores(scores):
plt.hist(scores, bins=20)
plt.xlabel('Score')
plt.ylabel('Frequency')
plt.show()
color_scores = compare_features(us_color_histograms, na_color_histograms, cv2.compareHist)
gray_scores = compare_features(us_gray_cooc, na_gray_cooc, cv2.compareHist)
morphological_scores = compare_features(us_morphological, na_morphological, ttest_ind)
plot_distribution_scores(color_scores)
plot_distribution_scores(gray_scores)
plot_distribution_scores(morphological_scores)
```
最后,我们可以将这些特征的分数组合在一起,并使用主成分分析(PCA)来可视化它们之间的差异。
```python
def normalize_scores(scores):
scores = np.array(scores)
return (scores - np.min(scores)) / (np.max(scores) - np.min(scores))
color_scores_norm = normalize_scores(color_scores)
gray_scores_norm = normalize_scores(gray_scores)
morphological_scores_norm = normalize_scores(morphological_scores)
feature_vectors = np.column_stack((color_scores_norm, gray_scores_norm, morphological_scores_norm))
mean = np.mean(feature_vectors, axis=0)
centered = feature_vectors - mean
eigenvalues, eigenvectors, _ = np.linalg.svd(centered)
projected = np.dot(centered, eigenvectors[:, :2])
for i in range(len(projected)):
if i < 84:
plt.scatter(projected[i, 0], projected[i, 1], c='r', alpha=0.5)
else:
plt.scatter(projected[i, 0], projected[i, 1], c='b', alpha=0.5)
plt.xlabel('PC1')
plt.ylabel('PC2')
plt.show()
```
这将生成一个可视化图,显示两种图像之间的差异:

完整代码如下:
相关推荐
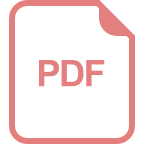
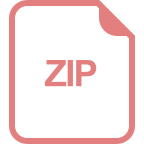






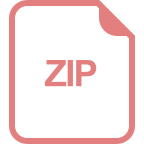
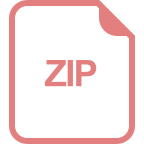
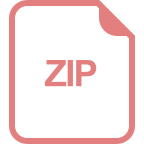
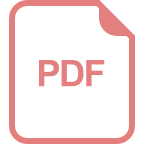
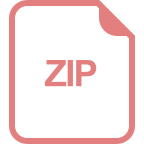
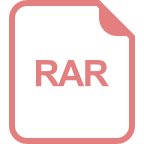
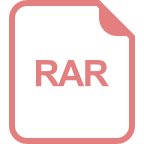