write a camera config stream program
时间: 2023-07-09 16:05:55 浏览: 98
To write a camera config stream program, you can follow the following steps:
1. Import the required libraries: You will need to import libraries like OpenCV, NumPy, and PiCamera for the camera configuration.
2. Configure the camera: Set up the camera configuration with the help of the PiCamera library. You can set up things like resolution, frames per second, and exposure settings.
3. Create a video stream: After configuring the camera, create a video stream using the OpenCV library. This stream will continuously capture frames from the camera and display them on the screen.
4. Display the video stream: Finally, display the video stream on the screen using the OpenCV library. You can also add some extra functionalities like image processing, object detection, and face recognition to the stream.
Here is a sample code that performs the above steps:
```python
import cv2
import numpy as np
from picamera import PiCamera
# Set up the camera configuration
camera = PiCamera()
camera.resolution = (640, 480)
camera.framerate = 32
# Create a video stream
video_stream = cv2.VideoCapture(0)
# Define the loop to capture frames from the camera
while True:
# Capture frame-by-frame
ret, frame = video_stream.read()
# Display the resulting frame
cv2.imshow('Video Stream', frame)
# Exit the loop if the user presses 'q'
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the capture and destroy all windows
video_stream.release()
cv2.destroyAllWindows()
```
This code will create a video stream that captures frames from the camera and displays them on the screen. The loop will continue until the user presses 'q'. You can modify this code to add extra functionalities like image processing, object detection, and face recognition.
相关推荐
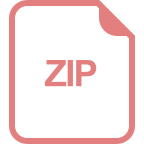
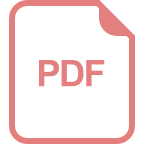
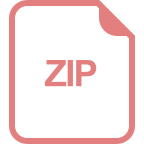














