任务描述 本关任务:编写程序实现节点类 Node,实现链表的基本操作。 编程要求 根据提示,在右侧编辑器补充 Node 类代码,实现 main 函数里调用的成员函数。
时间: 2024-05-15 13:13:12 浏览: 83
以下是 Node 类的代码示例:
```cpp
#include <iostream>
using namespace std;
class Node {
public:
int val;
Node* next;
Node(int _val) {
val = _val;
next = nullptr;
}
void add(Node* node) {
if (next == nullptr) {
next = node;
} else {
next->add(node);
}
}
void remove(Node* node) {
if (next == node) {
next = node->next;
} else if (next != nullptr) {
next->remove(node);
}
}
void print() {
cout << val << " ";
if (next != nullptr) {
next->print();
} else {
cout << endl;
}
}
};
int main() {
Node* head = new Node(1);
head->add(new Node(2));
head->add(new Node(3));
head->add(new Node(4));
head->print();
Node* node_to_remove = head->next->next;
head->remove(node_to_remove);
head->print();
return 0;
}
```
Node 类实现了链表的基本操作,包括添加节点、删除节点和打印链表。在 main 函数中,我们创建了一个链表并打印出来,然后删除了第三个节点并再次打印链表。
阅读全文
相关推荐
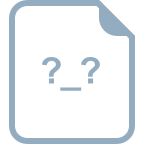
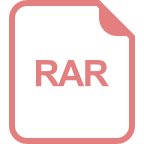





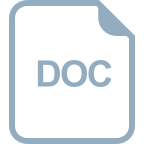
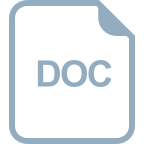
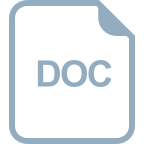
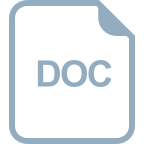
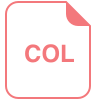
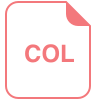
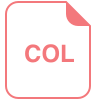



