C语言 利用链表实现一个简单的学生信息管理系统: (1)学生信息包括学号、姓名、性别、年龄、电话号码、所学专业、班级等; (2)系统的主要功能包括:学生信息的创建、输出学生信息、查询学生信息、增加学生信息、删除学生信息。
时间: 2024-12-02 16:21:58 浏览: 39
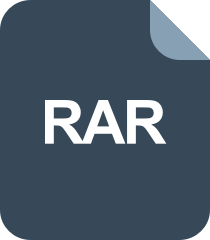
学生信息管理系统,实现学生信息包括:学号、姓名、性别、年龄、班级等信息。

在C语言中,我们可以利用链表数据结构来实现一个简单的学生信息管理系统。首先,我们需要定义一个结构体表示学生信息,然后创建一个链表节点和链表结构。
1. **定义学生信息结构体**:
```c
typedef struct {
int id; // 学号
char name[50]; // 姓名
char gender[10]; // 性别
int age; // 年龄
char phone[20]; // 电话号码
char major[50]; // 所学专业
char class_name[50];// 班级
struct Node *next; // 链接指针
} Student;
```
2. **定义链表节点结构**:
```c
typedef struct Node {
Student student_info;
struct Node *next;
} Node;
```
3. **链表类**:
```c
struct LinkedList {
Node *head;
};
```
4. **链表实现的功能函数**:
- **初始化链表**:
```c
void initList(LinkedList *list) {
list->head = NULL;
}
```
- **添加学生信息**:
```c
void addStudent(LinkedList *list, Student student) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->student_info = student;
newNode->next = list->head;
list->head = newNode;
}
```
- **输出所有学生信息**:
```c
void displayStudents(LinkedList *list) {
Node *current = list->head;
while (current != NULL) {
printf("学号:%d, 姓名:%s, ... \n", current->student_info.id, current->student_info.name);
current = current->next;
}
}
```
- **查询学生信息**:
```c
Student* findStudentById(LinkedList *list, int id) {
Node *current = list->head;
while (current != NULL) {
if (current->student_info.id == id) {
return ¤t->student_info;
}
current = current->next;
}
return NULL;
}
```
- **增加学生信息**:
```c
void addStudentInfo(Student *student, LinkedList *list) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->student_info = *student;
newNode->next = list->head;
list->head = newNode;
}
```
- **删除学生信息**:
```c
void deleteStudentById(LinkedList *list, int id) {
if (!list->head) return;
if (list->head->student_info.id == id) {
Node *temp = list->head;
list->head = list->head->next;
free(temp);
return;
}
Node *prev = list->head;
Node *current = list->head->next;
while (current != NULL && current->student_info.id != id) {
prev = current;
current = current->next;
}
if (current != NULL) {
prev->next = current->next;
free(current);
}
}
```
阅读全文
相关推荐
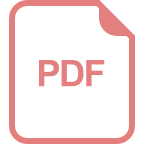
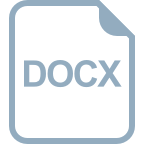














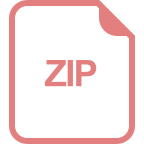
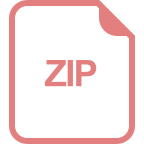
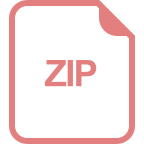