c语言数据结构利用链表实现一个简单的学生信息管理系统: (1)学生信息包括学号、姓名、性别、年龄、电话号码、所学专业、班级等; (2)系统的主要功能包括:学生信息的创建、输出学生信息、查询学生信息、增加学生信息、删除学生信息。
时间: 2024-11-13 10:27:12 浏览: 9
在C语言中,使用链表数据结构可以有效地实现一个简单的学生信息管理系统。首先,我们需要定义一个结构体(struct),用于存储学生的各项信息:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生信息结构体
typedef struct {
int id; // 学号
char name[50]; // 姓名
char gender[10]; // 性别
int age; // 年龄
char phone[20]; // 电话号码
char major[50]; // 所学专业
char class_name[50]; // 班级
} Student;
// 链表节点结构
typedef struct Node {
Student info;
struct Node* next;
} Node;
// 创建链表头指针
Node* head = NULL;
```
接下来,我们可以实现系统的主要功能:
1. **创建学生信息**:动态分配内存并初始化新节点的数据。
2. **输出学生信息**:遍历链表打印每个学生的详细信息。
3. **查询学生信息**:根据学号或其他关键字查找特定学生。
4. **增加学生信息**:在链表末尾插入新的学生节点。
5. **删除学生信息**:根据条件找到并移除指定的节点。
这里是一个简化版的功能实现示例,完整代码会包含更多的输入验证和错误处理:
```c
void createStudent() {
// ...获取用户输入,动态分配和初始化节点...
}
void displayStudents() {
Node* current = head;
while (current != NULL) {
printf("学号:%d 姓名:%s\n", current->info.id, current->info.name);
current = current->next;
}
}
Student* searchStudent(int id) {
Node* current = head;
while (current != NULL) {
if (current->info.id == id) return ¤t->info;
current = current->next;
}
return NULL;
}
void addStudent() {
// ...根据createStudent函数创建的新节点加入链表...
}
void removeStudent(int id) {
Node* current = head;
Node* previous = NULL;
while (current != NULL && current->info.id != id) {
previous = current;
current = current->next;
}
if (current != NULL) {
if (previous == NULL) head = current->next;
else previous->next = current->next;
free(current); // 释放节点内存
}
}
```
阅读全文
相关推荐
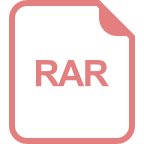
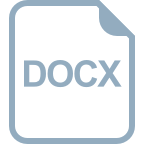
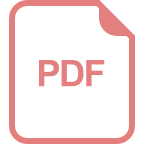










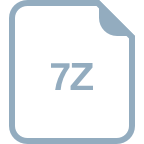
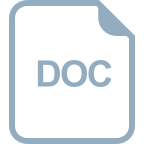
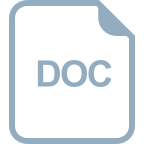

