两个GRBL连接Mega2560和迪文屏,以及通过迪文屏触摸控制命令驱动两个GRBL的代码
时间: 2024-04-30 21:18:14 浏览: 141
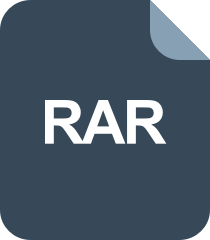
grbl-servo-master_两轴_grblservo_grbl-servo-master_写字机_写字机固件_

以下是通过迪文屏触摸控制命令驱动两个GRBL的代码:
```C++
#include <SoftwareSerial.h>
#include <Adafruit_GFX.h>
#include <Adafruit_ILI9341.h>
#include <TouchScreen.h>
#define TFT_CS 10
#define TFT_DC 9
#define TFT_RST 8
#define TS_CS 7
#define BAUDRATE 115200
// GRBL 1
SoftwareSerial grbl1Serial(2, 3); // RX, TX
bool grbl1Connected = false;
// GRBL 2
SoftwareSerial grbl2Serial(4, 5); // RX, TX
bool grbl2Connected = false;
// Touchscreen
TouchScreen ts = TouchScreen(XP, YP, XM, YM, 300);
// Display
Adafruit_ILI9341 tft = Adafruit_ILI9341(TFT_CS, TFT_DC, TFT_RST);
// Button
struct Button {
int x;
int y;
int width;
int height;
String text;
uint16_t color;
bool pressed;
};
// Buttons
Button btn1 = {
10, 10, 80, 40, "GRBL 1", ILI9341_RED, false
};
Button btn2 = {
100, 10, 80, 40, "GRBL 2", ILI9341_GREEN, false
};
Button btnHome = {
220, 10, 80, 40, "Home", ILI9341_BLUE, false
};
Button btnReset = {
220, 80, 80, 40, "Reset", ILI9341_YELLOW, false
};
Button btnStop = {
220, 150, 80, 40, "Stop", ILI9341_PURPLE, false
};
Button btnXPlus = {
10, 80, 40, 40, "+X", ILI9341_CYAN, false
};
Button btnXMinus = {
60, 80, 40, 40, "-X", ILI9341_CYAN, false
};
Button btnYPlus = {
110, 80, 40, 40, "+Y", ILI9341_CYAN, false
};
Button btnYMinus = {
160, 80, 40, 40, "-Y", ILI9341_CYAN, false
};
Button btnZPlus = {
210, 80, 40, 40, "+Z", ILI9341_CYAN, false
};
Button btnZMinus = {
260, 80, 40, 40, "-Z", ILI9341_CYAN, false
};
void setup() {
Serial.begin(BAUDRATE);
grbl1Serial.begin(BAUDRATE);
grbl2Serial.begin(BAUDRATE);
tft.begin();
tft.setRotation(1);
pinMode(TS_CS, OUTPUT);
ts.begin();
}
void loop() {
// Check if GRBL 1 is connected
if (!grbl1Connected) {
grbl1Serial.write("\r\n\r\n");
delay(1000);
while (grbl1Serial.available()) {
char c = grbl1Serial.read();
if (c == '\n') {
grbl1Connected = true;
break;
}
}
}
// Check if GRBL 2 is connected
if (!grbl2Connected) {
grbl2Serial.write("\r\n\r\n");
delay(1000);
while (grbl2Serial.available()) {
char c = grbl2Serial.read();
if (c == '\n') {
grbl2Connected = true;
break;
}
}
}
// Check for button press
TSPoint p = ts.getPoint();
pinMode(TS_CS, OUTPUT);
digitalWrite(TS_CS, LOW);
if (p.z > ts.pressureThreshhold) {
int x = map(p.x, TS_MINX, TS_MAXX, 0, tft.width());
int y = map(p.y, TS_MINY, TS_MAXY, 0, tft.height());
if (btn1.x <= x && x <= btn1.x + btn1.width &&
btn1.y <= y && y <= btn1.y + btn1.height) {
btn1.pressed = true;
} else if (btn2.x <= x && x <= btn2.x + btn2.width &&
btn2.y <= y && y <= btn2.y + btn2.height) {
btn2.pressed = true;
} else if (btnHome.x <= x && x <= btnHome.x + btnHome.width &&
btnHome.y <= y && y <= btnHome.y + btnHome.height) {
if (btn1.pressed) {
grbl1Serial.write("$H\n");
} else if (btn2.pressed) {
grbl2Serial.write("$H\n");
}
} else if (btnReset.x <= x && x <= btnReset.x + btnReset.width &&
btnReset.y <= y && y <= btnReset.y + btnReset.height) {
if (btn1.pressed) {
grbl1Serial.write(String((char)0x18) + "\n");
} else if (btn2.pressed) {
grbl2Serial.write(String((char)0x18) + "\n");
}
} else if (btnStop.x <= x && x <= btnStop.x + btnStop.width &&
btnStop.y <= y && y <= btnStop.y + btnStop.height) {
if (btn1.pressed) {
grbl1Serial.write(String((char)0x85) + "\n");
} else if (btn2.pressed) {
grbl2Serial.write(String((char)0x85) + "\n");
}
} else if (btnXPlus.x <= x && x <= btnXPlus.x + btnXPlus.width &&
btnXPlus.y <= y && y <= btnXPlus.y + btnXPlus.height) {
if (btn1.pressed) {
grbl1Serial.write("G91 G0 X10\n");
} else if (btn2.pressed) {
grbl2Serial.write("G91 G0 X10\n");
}
} else if (btnXMinus.x <= x && x <= btnXMinus.x + btnXMinus.width &&
btnXMinus.y <= y && y <= btnXMinus.y + btnXMinus.height) {
if (btn1.pressed) {
grbl1Serial.write("G91 G0 X-10\n");
} else if (btn2.pressed) {
grbl2Serial.write("G91 G0 X-10\n");
}
} else if (btnYPlus.x <= x && x <= btnYPlus.x + btnYPlus.width &&
btnYPlus.y <= y && y <= btnYPlus.y + btnYPlus.height) {
if (btn1.pressed) {
grbl1Serial.write("G91 G0 Y10\n");
} else if (btn2.pressed) {
grbl2Serial.write("G91 G0 Y10\n");
}
} else if (btnYMinus.x <= x && x <= btnYMinus.x + btnYMinus.width &&
btnYMinus.y <= y && y <= btnYMinus.y + btnYMinus.height) {
if (btn1.pressed) {
grbl1Serial.write("G91 G0 Y-10\n");
} else if (btn2.pressed) {
grbl2Serial.write("G91 G0 Y-10\n");
}
} else if (btnZPlus.x <= x && x <= btnZPlus.x + btnZPlus.width &&
btnZPlus.y <= y && y <= btnZPlus.y + btnZPlus.height) {
if (btn1.pressed) {
grbl1Serial.write("G91 G0 Z10\n");
} else if (btn2.pressed) {
grbl2Serial.write("G91 G0 Z10\n");
}
} else if (btnZMinus.x <= x && x <= btnZMinus.x + btnZMinus.width &&
btnZMinus.y <= y && y <= btnZMinus.y + btnZMinus.height) {
if (btn1.pressed) {
grbl1Serial.write("G91 G0 Z-10\n");
} else if (btn2.pressed) {
grbl2Serial.write("G91 G0 Z-10\n");
}
}
} else {
if (btn1.pressed) {
btn1.pressed = false;
} else if (btn2.pressed) {
btn2.pressed = false;
}
}
// Draw buttons
tft.fillScreen(ILI9341_BLACK);
tft.drawRect(btn1.x, btn1.y, btn1.width, btn1.height, btn1.color);
tft.setCursor(btn1.x + 10, btn1.y + btn1.height / 2 - 4);
tft.print(btn1.text);
tft.drawRect(btn2.x, btn2.y, btn2.width, btn2.height, btn2.color);
tft.setCursor(btn2.x + 10, btn2.y + btn2.height / 2 - 4);
tft.print(btn2.text);
tft.drawRect(btnHome.x, btnHome.y, btnHome.width, btnHome.height, btnHome.color);
tft.setCursor(btnHome.x + 10, btnHome.y + btnHome.height / 2 - 4);
tft.print(btnHome.text);
tft.drawRect(btnReset.x, btnReset.y, btnReset.width, btnReset.height, btnReset.color);
tft.setCursor(btnReset.x + 10, btnReset.y + btnReset.height / 2 - 4);
tft.print(btnReset.text);
tft.drawRect(btnStop.x, btnStop.y, btnStop.width, btnStop.height, btnStop.color);
tft.setCursor(btnStop.x + 10, btnStop.y + btnStop.height / 2 - 4);
tft.print(btnStop.text);
tft.drawRect(btnXPlus.x, btnXPlus.y, btnXPlus.width, btnXPlus.height, btnXPlus.color);
tft.setCursor(btnXPlus.x + 10, btnXPlus.y + btnXPlus.height / 2 - 4);
tft.print(btnXPlus.text);
tft.drawRect(btnXMinus.x, btnXMinus.y, btnXMinus.width, btnXMinus.height, btnXMinus.color);
tft.setCursor(btnXMinus.x + 10, btnXMinus.y + btnXMinus.height / 2 - 4);
tft.print(btnXMinus.text);
tft.drawRect(btnYPlus.x, btnYPlus.y, btnYPlus.width, btnYPlus.height, btnYPlus.color);
tft.setCursor(btnYPlus.x + 10, btnYPlus.y + btnYPlus.height / 2 - 4);
tft.print(btnYPlus.text);
tft.drawRect(btnYMinus.x, btnYMinus.y, btnYMinus.width, btnYMinus.height, btnYMinus.color);
tft.setCursor(btnYMinus.x + 10, btnYMinus.y + btnYMinus.height / 2 - 4);
tft.print(btnYMinus.text);
tft.drawRect(btnZPlus.x, btnZPlus.y, btnZPlus.width, btnZPlus.height, btnZPlus.color);
tft.setCursor(btnZPlus.x + 10, btnZPlus.y + btnZPlus.height / 2 - 4);
tft.print(btnZPlus.text);
tft.drawRect(btnZMinus.x, btnZMinus.y, btnZMinus.width, btnZMinus.height, btnZMinus.color);
tft.setCursor(btnZMinus.x + 10, btnZMinus.y + btnZMinus.height / 2 - 4);
tft.print(btnZMinus.text);
// Read from GRBL 1
while (grbl1Serial.available()) {
char c = grbl1Serial.read();
Serial.write(c);
}
// Read from GRBL 2
while (grbl2Serial.available()) {
char c = grbl2Serial.read();
Serial.write(c);
}
}
```
该代码使用了 Adafruit_GFX 库和 Adafruit_ILI9341 库来控制迪文屏,使用了 TouchScreen 库来获取触摸屏输入,使用了 SoftwareSerial 库来实现与两个 GRBL 的通信。
在 setup() 函数中,所有的串口都被初始化,并且迪文屏和触摸屏被设置为正常工作。
在 loop() 函数中,首先检查两个 GRBL 是否连接。如果未连接,则发送一些字符来检查它们是否回应。如果回应,则认为它们已连接。
然后,检查触摸屏是否有按钮按下。如果是,则根据按下的按钮发送相应的 G-code 命令到 GRBL。如果不是,则检查之前是否有按钮按下,如果是,则将其重置为未按下状态。最后,绘制所有按钮并检查 GRBL 的串口是否有数据,如果有,则将其打印到串口监视器上。
这是一个基本的代码示例,可以根据实际情况进行修改和扩展。
阅读全文
相关推荐
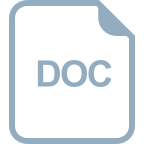
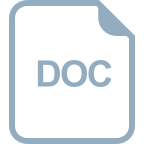
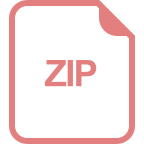
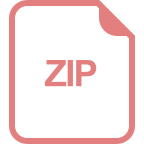
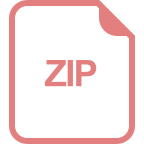
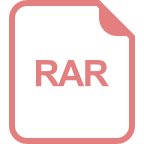
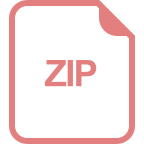
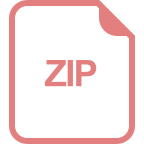
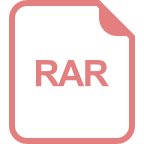
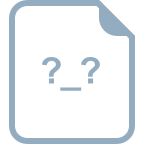
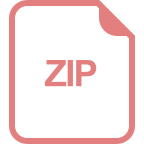
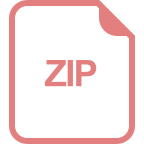
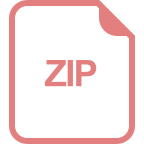



