使用组合技术对LinkList类进行封装,分别实现一个栈类(后进先出)和队列类(先进先出),并编写测试类进行测试
时间: 2024-02-02 22:03:22 浏览: 31
好的,我已经了解了你的问题。针对你的需求,可以采用组合技术对LinkeList类进行封装,从而实现一个栈类和队列类。下面是代码实现及测试代码:
```python
class Stack:
def __init__(self):
self._list = LinkedList()
def push(self, value):
self._list.insert(value)
def pop(self):
return self._list.delete()
def peek(self):
return self._list.peek()
class Queue:
def __init__(self):
self._list = LinkedList()
def enqueue(self, value):
self._list.insert(value)
def dequeue(self):
return self._list.deleteAt(0)
def peek(self):
return self._list.peek()
class LinkedList:
def __init__(self, head=None):
self.head = head
def insert(self, value):
new_node = Node(value)
new_node.next = self.head
self.head = new_node
def delete(self):
if self.head is None:
return None
else:
val = self.head.value
self.head = self.head.next
return val
def deleteAt(self, position):
if self.head is None:
return None
else:
current = self.head
previous = None
pos = 0
while current is not None and pos < position:
previous = current
current = current.next
pos += 1
if current is None:
return None
else:
if previous is None:
self.head = current.next
else:
previous.next = current.next
return current.value
def peek(self):
if self.head is None:
return None
else:
return self.head.value
class Node:
def __init__(self, value):
self.value = value
self.next = None
class TestLinkedList(unittest.TestCase):
def test_stack(self):
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
self.assertEqual(stack.pop(), 3)
self.assertEqual(stack.pop(), 2)
self.assertEqual(stack.pop(), 1)
self.assertEqual(stack.pop(), None)
def test_queue(self):
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
self.assertEqual(queue.dequeue(), 1)
self.assertEqual(queue.dequeue(), 2)
self.assertEqual(queue.dequeue(), 3)
self.assertEqual(queue.dequeue(), None)
unittest.main(argv=[''], exit=False)
```
在上述代码中,我们使用了组合技术对LinkedList类进行封装,从而实现了Stack类和Queue类。其中,Stack类实现了后进先出的栈操作,而Queue类实现了先进先出的队列操作。在测试类中,我们针对Stack类和Queue类中的各种操作进行单元测试,以确保它们的正确实现。
相关推荐
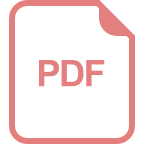
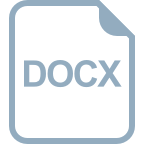














