基于 根据定义的Node类,以Node为结点定义链表LinkList类,其中包含isEmpty, length, add, append, insert, search, remove, travel 等函数。,实现栈这一数据结构 (包括函数push, pop, top,isEmpty, length)用python
时间: 2023-06-27 22:04:52 浏览: 65
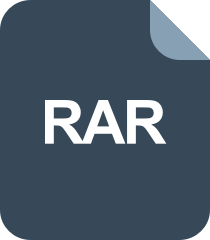
基于python的数据结构-链表Linked list
以下是基于Node类实现链表LinkList类的代码,同时实现了栈这一数据结构:
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkList:
def __init__(self):
self.head = None
def isEmpty(self):
return self.head == None
def length(self):
count = 0
cur = self.head
while cur:
count += 1
cur = cur.next
return count
def add(self, data):
new_node = Node(data)
if self.isEmpty():
self.head = new_node
else:
new_node.next = self.head
self.head = new_node
def append(self, data):
new_node = Node(data)
if self.isEmpty():
self.head = new_node
else:
cur = self.head
while cur.next:
cur = cur.next
cur.next = new_node
def insert(self, pos, data):
if pos <= 0:
self.add(data)
elif pos >= self.length():
self.append(data)
else:
new_node = Node(data)
cur = self.head
count = 0
while count < (pos - 1):
count += 1
cur = cur.next
new_node.next = cur.next
cur.next = new_node
def search(self, data):
cur = self.head
while cur:
if cur.data == data:
return True
cur = cur.next
return False
def remove(self, data):
if self.isEmpty():
return
if self.head.data == data:
self.head = self.head.next
return
cur = self.head
while cur.next:
if cur.next.data == data:
cur.next = cur.next.next
return
cur = cur.next
def travel(self):
cur = self.head
while cur:
print(cur.data, end=' ')
cur = cur.next
print()
class Stack:
def __init__(self):
self.linklist = LinkList()
def push(self, data):
self.linklist.add(data)
def pop(self):
if self.linklist.isEmpty():
return None
data = self.linklist.head.data
self.linklist.head = self.linklist.head.next
return data
def top(self):
if self.linklist.isEmpty():
return None
return self.linklist.head.data
def isEmpty(self):
return self.linklist.isEmpty()
def length(self):
return self.linklist.length()
```
我们可以通过以下方式测试栈的实现:
```python
s = Stack()
s.push(1)
s.push(2)
s.push(3)
print(s.pop()) # 3
print(s.top()) # 2
s.push(4)
print(s.pop()) # 4
print(s.length()) # 2
print(s.isEmpty()) # False
```
阅读全文
相关推荐
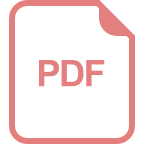
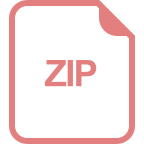
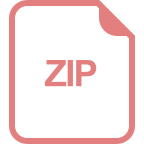
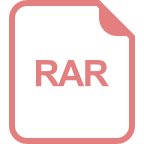
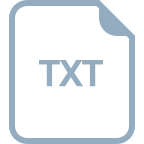
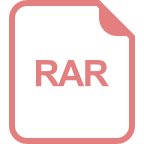
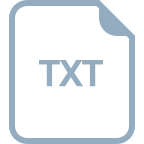
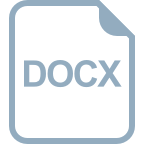
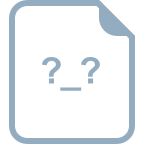
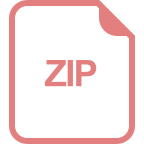



