c++编写程序实现节点类 Node,实现链表的基本操作
时间: 2023-08-16 20:07:04 浏览: 47
好的,根据你的要求,我可以为你编写一段 C++ 代码来实现节点类 Node,并实现链表的基本操作。下面是代码:
```
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int val) {
data = val;
next = nullptr;
}
};
class LinkedList {
private:
Node* head;
int size;
public:
LinkedList() {
head = nullptr;
size = 0;
}
void addFirst(int val) {
Node* node = new Node(val);
node->next = head;
head = node;
size++;
}
void addLast(int val) {
Node* node = new Node(val);
if (head == nullptr) {
head = node;
} else {
Node* cur = head;
while (cur->next != nullptr) {
cur = cur->next;
}
cur->next = node;
}
size++;
}
void removeFirst() {
if (head != nullptr) {
Node* temp = head;
head = head->next;
delete temp;
size--;
}
}
void removeLast() {
if (head != nullptr) {
if (head->next == nullptr) {
delete head;
head = nullptr;
} else {
Node* cur = head;
while (cur->next->next != nullptr) {
cur = cur->next;
}
delete cur->next;
cur->next = nullptr;
}
size--;
}
}
void display() {
Node* cur = head;
while (cur != nullptr) {
cout << cur->data << " ";
cur = cur->next;
}
cout << endl;
}
int getSize() {
return size;
}
};
int main() {
LinkedList list;
list.addLast(3);
list.addLast(1);
list.addLast(4);
list.addLast(8);
list.addLast(6);
list.display(); // 输出:3 1 4 8 6
list.removeFirst();
list.removeLast();
list.display(); // 输出:1 4 8
return 0;
}
```
这段代码定义了一个节点类 Node,以及一个链表类 LinkedList,并实现了链表的基本操作,包括在链表头部添加节点、在链表尾部添加节点、删除链表头部节点、删除链表尾部节点、打印链表元素等。在 main 函数中使用 LinkedList 对象测试了这些操作的正确性。
相关推荐
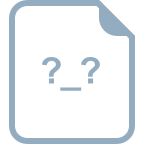
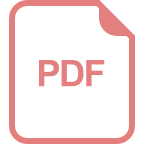
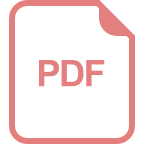














