用C++编写程序:编写两个函数,实现动态链表的建立和输出,主函数调用。
时间: 2024-03-22 20:38:56 浏览: 79
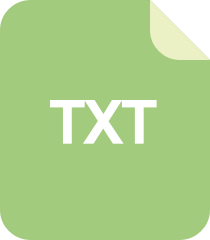
C++编写链表程序
好的,下面是代码示例:
```c++
#include <iostream>
using namespace std;
// 定义链表结构体
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
// 建立链表
ListNode* createList() {
ListNode *head = new ListNode(0); // 创建头结点
ListNode *p = head; // 定义指针p,初始指向头结点
int n;
cout << "请输入链表长度:";
cin >> n;
cout << "请输入链表元素:";
for (int i = 0; i < n; i++) {
int val;
cin >> val;
ListNode *node = new ListNode(val); // 创建新节点
p->next = node; // 将新节点插入到链表尾部
p = node; // p指向新节点
}
return head->next; // 返回头结点的下一个节点,即链表的第一个节点
}
// 输出链表
void printList(ListNode *head) {
ListNode *p = head;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
int main() {
ListNode *head = createList(); // 建立链表
printList(head); // 输出链表
return 0;
}
```
主函数调用了两个函数,分别是 `createList` 和 `printList`。`createList` 函数用来建立链表,`printList` 函数用来输出链表。其中,`ListNode` 是链表的结构体,包含一个整型变量 `val` 和一个指针变量 `next`,`createList` 函数中的 `for` 循环用来读入链表元素,`printList` 函数中的 `while` 循环用来遍历链表并输出链表元素。
阅读全文
相关推荐




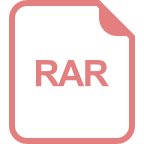
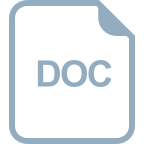
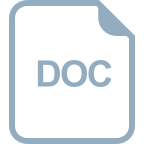
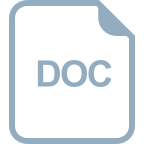







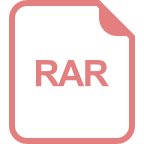