用c语言定义结点类型,创建链表,遍历链表
时间: 2023-12-12 13:54:27 浏览: 79
下面是一个简单的例子,用C语言定义结点类型,创建链表,遍历链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data; // 数据域
struct Node* next; // 指针域
};
// 创建链表函数
struct Node* createLinkedList(int n) {
struct Node* head = NULL;
struct Node* tail = NULL;
for (int i = 1; i <= n; i++) {
// 创建一个新的节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = i;
newNode->next = NULL;
if (head == NULL) {
// 如果链表为空,则新节点为头节点和尾节点
head = newNode;
tail = newNode;
} else {
// 否则,将新节点插入到尾节点后面
tail->next = newNode;
tail = newNode;
}
}
return head;
}
// 遍历链表函数
void traverseLinkedList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
// 创建链表
struct Node* head = createLinkedList(5);
// 遍历链表
traverseLinkedList(head);
return 0;
}
```
在这个例子中,我们定义了一个 `Node` 结构体来表示链表的节点,包含一个整数数据域和一个指向下一个节点的指针域。`createLinkedList` 函数用于创建一个包含 `n` 个节点的链表,并返回链表的头节点。`traverseLinkedList` 函数用于遍历链表并输出每个节点的数据。在 `main` 函数中,我们调用 `createLinkedList` 创建了一个包含5个节点的链表,并调用 `traverseLinkedList` 遍历输出链表中的数据。
阅读全文
相关推荐


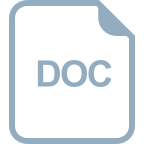

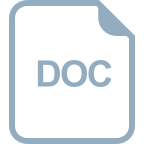
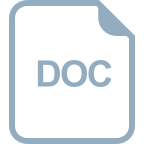









