写一个贪心算法的路程Java程序
时间: 2023-07-10 17:30:02 浏览: 81
下面是一个简单的贪心算法的Java程序,该程序用于求解旅行商问题(TSP)。在这个问题中,有n个城市需要旅行商依次访问,每个城市之间的距离已知。要求旅行商从某个起点出发,依次访问所有城市一次,最后回到起点。求访问所有城市的最短路径长度。
```java
import java.util.Arrays;
class TSP {
// 贪心算法求解旅行商问题
public static int tsp(int[][] graph) {
int n = graph.length;
boolean[] visited = new boolean[n];
Arrays.fill(visited, false);
visited[0] = true;
int current = 0;
int cost = 0;
for (int i = 1; i < n; i++) {
int next = -1;
int minCost = Integer.MAX_VALUE;
for (int j = 0; j < n; j++) {
if (!visited[j] && graph[current][j] < minCost) {
minCost = graph[current][j];
next = j;
}
}
if (next == -1) {
// 无法找到下一个未访问的城市
return -1;
}
cost += minCost;
visited[next] = true;
current = next;
}
cost += graph[current][0]; // 回到起点
return cost;
}
public static void main(String[] args) {
int[][] graph = {
{0, 2, 9, 10},
{1, 0, 6, 4},
{15, 7, 0, 8},
{6, 3, 12, 0}
};
int result = tsp(graph);
System.out.println("最短路径长度为:" + result);
}
}
```
该程序实现了一个贪心算法,用于求解旅行商问题(TSP)。它从起点出发,依次选择距离最近的未访问城市进行访问,直到所有城市都被访问过。程序输出访问所有城市的最短路径长度。
阅读全文
相关推荐
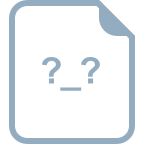
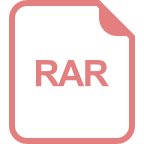
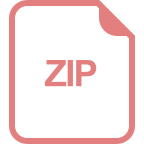
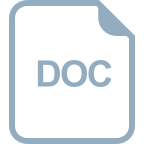
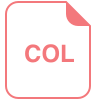



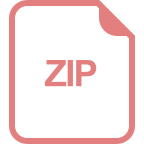
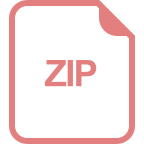
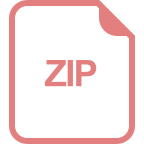
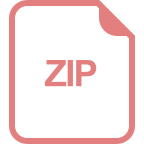