给我用Java 写出一个贪心算法
时间: 2023-05-13 13:07:44 浏览: 44
我可以给你写一个贪心算法的Java程序:
public class GreedyAlgorithm {
public static void main(String[] args) {
int[] arr = {7, 10, 12, 15, 20};
int sum = 0;
int i = 0;
while (i < arr.length) {
if (arr[i] + sum <= 20) {
sum += arr[i];
}
i++;
}
System.out.println("最大和为:" + sum);
}
}
相关问题
用java写一个贪心算法
好的,以下是一个使用 Java 编写的贪心算法示例:
```
import java.util.Arrays;
public class GreedyAlgorithm {
public static void main(String[] args) {
int[] coins = {1, 5, 10, 25}; // 硬币面值
int amount = 63; // 需要凑出的金额
int[] usedCoins = new int[coins.length]; // 记录每种硬币使用的数量
// 从大到小遍历硬币面值
for (int i = coins.length - 1; i >= 0; i--) {
int coin = coins[i];
while (amount >= coin) { // 如果金额大于等于当前硬币面值
amount -= coin; // 减去当前硬币面值
usedCoins[i]++; // 记录当前硬币使用数量
}
}
// 输出结果
System.out.println("需要的硬币数量:" + Arrays.stream(usedCoins).sum());
for (int i = 0; i < coins.length; i++) {
System.out.println(coins[i] + " 元硬币使用了 " + usedCoins[i] + " 个");
}
}
}
```
这个程序实现了一个简单的贪心算法,用最少的硬币凑出指定的金额。
用java写一个最短路径算法
### 回答1:
您可以使用 Dijkstra 算法来解决最短路径问题。该算法基于贪心策略,通过不断地选择未确定的最短路径节点来实现。在 Java 中,您可以使用优先队列来维护未确定的节点,从而确定最短路径。
以下是 Java 代码的简单实现:
```
import java.util.*;
class Dijkstra {
private int dist[];
private Set<Integer> settled;
private PriorityQueue<Node> pq;
private int V; // Number of vertices
List<List<Node>> adj;
public Dijkstra(int V) {
this.V = V;
dist = new int[V];
settled = new HashSet<Integer>();
pq = new PriorityQueue<Node>(V, new Node());
}
// Function for Dijkstra's Algorithm
public void dijkstra(List<List<Node>> adj, int src) {
this.adj = adj;
for (int i = 0; i < V; i++)
dist[i] = Integer.MAX_VALUE;
// Add source node to the priority queue
pq.add(new Node(src, 0));
// Distance to the source is 0
dist[src] = 0;
while (settled.size() != V) {
// remove the minimum distance node
// from the priority queue
int u = pq.remove().node;
// adding the node whose distance is
// finalized
settled.add(u);
e_Neighbours(u);
}
}
// Function to process all the neighbours
// of the passed node
private void e_Neighbours(int u) {
int edgeDistance = -1;
int newDistance = -1;
// All the neighbors of v
for (int i = 0; i < adj.get(u).size(); i++) {
Node v = adj.get(u).get(i);
// If current node hasn't already been processed
if (!settled.contains(v.node)) {
edgeDistance = v.cost;
newDistance = dist[u] + edgeDistance;
// If new distance is cheaper in cost
if (newDistance < dist[v.node])
dist[v.node] = newDistance;
// Add the current node to the queue
pq.add(new Node(v.node, dist[v.node]));
}
}
}
// Driver code
public static void main(String arg[]) {
int V = 5;
int source =
### 回答2:
最短路径算法是一种计算图中两个节点之间最短路径的算法。常见的最短路径算法有Dijkstra算法和Floyd-Warshall算法。
在Java中,我们可以使用Dijkstra算法来实现最短路径的计算。首先,我们需要定义一个图的数据结构,用来存储节点以及节点之间的边。可以使用邻接矩阵或邻接表来表示图。接下来,我们可以使用Java的优先队列来实现Dijkstra算法。
算法步骤如下:
1. 创建一个优先队列和一个距离数组,用于存储节点到起始节点的最短距离。将起始节点的距离设置为0,其他节点的距离设置为无限大,并将起始节点加入优先队列。
2. 循环直到优先队列为空:
a. 弹出队列中距离最小的节点;
b. 遍历该节点的邻接节点,如果通过当前节点到达邻接节点的距离小于目前存储的距离,则更新距离数组,并将邻接节点加入优先队列。
3. 最终,距离数组中存储了每个节点到起始节点的最短路径距离。
以下是一个简单的使用Java实现Dijkstra算法的示例代码:
```java
import java.util.*;
public class Dijkstra {
public static void main(String[] args) {
int[][] graph = { { 0, 4, 0, 0, 0, 0, 0, 8, 0 },
{ 4, 0, 8, 0, 0, 0, 0, 11, 0 },
{ 0, 8, 0, 7, 0, 4, 0, 0, 2 },
{ 0, 0, 7, 0, 9, 14, 0, 0, 0 },
{ 0, 0, 0, 9, 0, 10, 0, 0, 0 },
{ 0, 0, 4, 14, 10, 0, 2, 0, 0 },
{ 0, 0, 0, 0, 0, 2, 0, 1, 6 },
{ 8, 11, 0, 0, 0, 0, 1, 0, 7 },
{ 0, 0, 2, 0, 0, 0, 6, 7, 0 } };
int[] shortestDistances = dijkstra(graph, 0);
System.out.println("起始节点到其他节点的最短路径距离:");
for (int i = 0; i < shortestDistances.length; i++) {
System.out.println("节点 " + i + " 的最短路径距离为:" + shortestDistances[i]);
}
}
public static int[] dijkstra(int[][] graph, int start) {
int n = graph.length;
int[] distances = new int[n];
Arrays.fill(distances, Integer.MAX_VALUE);
distances[start] = 0;
PriorityQueue<Node> pq = new PriorityQueue<>(Comparator.comparingInt(a -> a.distance));
pq.add(new Node(start, 0));
while (!pq.isEmpty()) {
Node current = pq.poll();
int currentNode = current.node;
int currentDistance = current.distance;
if (currentDistance > distances[currentNode]) {
continue;
}
for (int i = 0; i < n; i++) {
if (graph[currentNode][i] != 0) {
int newDistance = currentDistance + graph[currentNode][i];
if (newDistance < distances[i]) {
distances[i] = newDistance;
pq.add(new Node(i, newDistance));
}
}
}
}
return distances;
}
static class Node {
int node;
int distance;
public Node(int node, int distance) {
this.node = node;
this.distance = distance;
}
}
}
```
该示例代码使用邻接矩阵表示图,并计算了起始节点到其他节点的最短路径距离。
### 回答3:
最短路径算法是一种用于在图中找到两个节点之间最短路径的算法。其中最著名的算法之一是Dijkstra算法。下面是用Java语言编写Dijkstra算法的一个简单实现:
```java
import java.util.*;
public class ShortestPathAlgorithm {
public void dijkstra(int[][] graph, int startVertex) {
int numVertices = graph[0].length;
int[] shortestDistances = new int[numVertices];
boolean[] visited = new boolean[numVertices];
for (int i = 0; i < numVertices; i++) {
shortestDistances[i] = Integer.MAX_VALUE;
visited[i] = false;
}
shortestDistances[startVertex] = 0;
for (int i = 0; i < numVertices - 1; i++) {
int minDistanceVertex = findMinDistanceVertex(shortestDistances, visited);
visited[minDistanceVertex] = true;
for (int j = 0; j < numVertices; j++) {
if (!visited[j] && graph[minDistanceVertex][j] != 0 && shortestDistances[minDistanceVertex] != Integer.MAX_VALUE && shortestDistances[minDistanceVertex] + graph[minDistanceVertex][j] < shortestDistances[j]) {
shortestDistances[j] = shortestDistances[minDistanceVertex] + graph[minDistanceVertex][j];
}
}
}
// 输出最短路径
for (int i = 0; i < numVertices; i++) {
System.out.println("从起始节点到节点 " + i + " 的最短路径距离为: " + shortestDistances[i]);
}
}
private int findMinDistanceVertex(int[] shortestDistances, boolean[] visited) {
int minDistance = Integer.MAX_VALUE;
int minDistanceVertex = -1;
for (int i = 0; i < shortestDistances.length; i++) {
if (!visited[i] && shortestDistances[i] < minDistance) {
minDistance = shortestDistances[i];
minDistanceVertex = i;
}
}
return minDistanceVertex;
}
public static void main(String[] args) {
int[][] graph = {
{0, 4, 0, 0, 0, 0, 0, 8, 0},
{4, 0, 8, 0, 0, 0, 0, 11, 0},
{0, 8, 0, 7, 0, 4, 0, 0, 2},
{0, 0, 7, 0, 9, 14, 0, 0, 0},
{0, 0, 0, 9, 0, 10, 0, 0, 0},
{0, 0, 4, 14, 10, 0, 2, 0, 0},
{0, 0, 0, 0, 0, 2, 0, 1, 6},
{8, 11, 0, 0, 0, 0, 1, 0, 7},
{0, 0, 2, 0, 0, 0, 6, 7, 0}
};
ShortestPathAlgorithm shortestPathAlgorithm = new ShortestPathAlgorithm();
shortestPathAlgorithm.dijkstra(graph, 0);
}
}
```
在这个实现中,我们首先创建一个数组`shortestDistances`,其中存储着从起始节点到各个节点的最短路径距离。我们还创建一个布尔类型的数组`visited`,用于标记已访问过的节点。
然后,我们将起始节点的最短路径距离设置为0,并遍历图中的所有节点。对于每个节点,我们选择一个最短路径距离最小的节点,并将其标记为已访问。之后,我们检查当前节点与其相邻节点之间的路径是否产生了更短的路径,如果是的话,我们更新这些节点的最短路径距离。
最后,我们打印出从起始节点到每个节点的最短路径距离。
上述代码中给出了一个示例图,你可以修改此图或定义自己的图来测试该算法的功能。
相关推荐
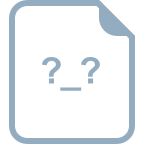
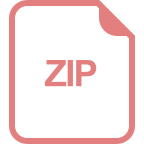













