将一张图像切分成为四份,在训练和测试时输入网络中。当四张都没有缺陷,则被认为是正常,反之则被认定为缺陷,切片操作,图像为1024*1024,划分成512*512 代码 pytorch
时间: 2024-04-29 08:21:39 浏览: 20
下面是一个简单的 PyTorch 代码实现,用于将图像切分为四个子图像,并使用它们来进行预测:
```python
import torch
import torch.nn as nn
import torchvision.transforms.functional as TF
from PIL import Image
def split_image(image):
# 切分图像为四个子图像
w, h = image.size
sub_images = []
for i in range(2):
for j in range(2):
left = i * w // 2
top = j * h // 2
right = (i + 1) * w // 2
bottom = (j + 1) * h // 2
sub_images.append(TF.crop(image, top, left, bottom-top, right-left))
return sub_images
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 16, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(16, 32, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(32, 64, kernel_size=3, padding=1)
self.fc1 = nn.Linear(64 * 64 * 64, 256)
self.fc2 = nn.Linear(256, 2)
def forward(self, x):
x = torch.cat([self.conv1(xi) for xi in x], dim=1)
x = F.relu(x)
x = F.max_pool2d(x, 2)
x = torch.cat([self.conv2(xi) for xi in x], dim=1)
x = F.relu(x)
x = F.max_pool2d(x, 2)
x = torch.cat([self.conv3(xi) for xi in x], dim=1)
x = F.relu(x)
x = F.max_pool2d(x, 2)
x = x.view(-1, 64 * 64 * 64)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
# 加载模型
model = Net()
model.load_state_dict(torch.load("model.pt"))
# 加载测试图像并进行预测
image = Image.open("test.jpg")
sub_images = split_image(image)
with torch.no_grad():
inputs = [TF.to_tensor(xi).unsqueeze(0) for xi in sub_images]
outputs = model(inputs)
preds = torch.argmax(outputs, dim=1)
if preds.sum() == 0:
print("正常")
else:
print("有缺陷")
```
需要注意的是,这只是一个简单的示例代码,您可能需要根据您的具体需求进行修改。此外,还需要定义训练数据集和训练过程,以便训练模型并保存模型参数。
相关推荐
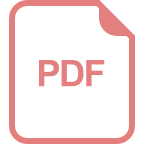
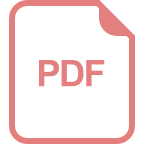
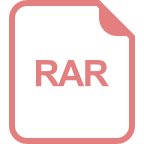


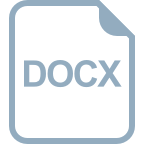
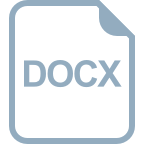
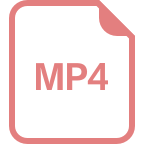
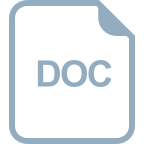