python统计字符串中各个字符
时间: 2024-10-04 12:04:53 浏览: 52
在Python中,你可以使用内置的`collections`模块中的`Counter`类来统计字符串中各个字符的出现次数。`Counter`是一个字典子类,用于计算元素出现的频率。下面是一个简单的例子:
```python
from collections import Counter
string = "hello world"
char_count = Counter(string)
print(char_count)
```
运行上述代码后,`char_count`将是一个字典,其中键是字符串中的字符,值是它们在字符串中出现的次数。例如,输出可能是`{'h': 1, 'e': 1, 'l': 3, 'o': 2, ' ': 1, 'w': 1, 'r': 1, 'd': 1}`。
如果你想获取每个字符及其出现次数的列表,可以这样做:
```python
char_list = list(char_count.items())
char_list.sort() # 如果需要按字母顺序排序
for char, freq in char_list:
print(f"字符 '{char}': 出现 {freq} 次")
```
相关问题
PYTHON统计字符串中各个字母出现的次数
可以使用Python中的字典来统计字符串中各个字母出现的次数。具体实现代码如下:
```python
str = 'hello world'
counts = {}
# 遍历字符串中的每个字母,并统计其出现次数
for char in str:
if char in counts:
counts[char] += 1
else:
counts[char] = 1
# 输出统计结果
for key, value in counts.items():
print(key, ':', value)
```
运行代码后,输出结果如下:
```
h : 1
e : 1
l : 3
o : 2
: 1
w : 1
r : 1
d : 1
```
python统计字符串中字母出现次数
您好!在Python统计字符串中字母出现次数,可以使用Python内置的collections模块中的Counter类,例如:
```python
from collections import Counter
# 定义字符串
s = "Hello World"
# 将字符串转换成小写,并去除空格
s = s.lower().replace(" ", "")
# 使用Counter类统计字母出现次数
count = Counter(s)
# 打印结果
for letter, frequency in count.items():
print(letter, frequency)
```
输出结果如下:
```
h 1
e 1
l 3
o 2
w 1
r 1
d 1
```
如上代码,通过将字符串转换为小写字母并去除空格,可以统计出字符串中各个字母出现的次数,最后使用for循环打印结果。其中,count.items()方法可以获取字典中的键值对,方便遍历打印结果。
阅读全文
相关推荐
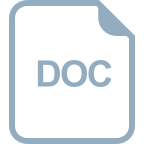
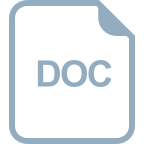
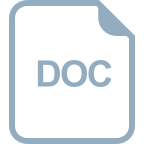
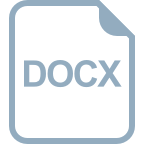
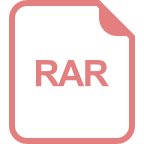











