51单片机和stm32进行串口通信,使用 51 单片机的一个按键k1,当按键k1按下时,stm32 上的一个 LED 灯点亮,5s 后自动熄灭。51和32代码分别是什么
时间: 2024-01-22 16:21:37 浏览: 72
以下是 51 单片机的代码:
```c
#include <reg52.h>
sbit K1 = P1^0; // 定义按键连接的引脚
sbit LED = P1^1; // 定义 LED 连接的引脚
void delay(unsigned int i) // 延时函数
{
while(i--);
}
void main()
{
TMOD = 0x20; // 设置定时器1为模式2
TH1 = 0xFD; // 定时器初值,5ms
TL1 = 0xFD;
TR1 = 1; // 启动定时器1
ET1 = 1; // 允许定时器1中断
EA = 1; // 全局中断允许
while(1)
{
if(!K1) // 检测按键是否按下
{
LED = 1; // 点亮 LED
while(!K1); // 等待按键释放
delay(1000); // 延时 5s
LED = 0; // 熄灭 LED
}
}
}
void timer1() interrupt 3 // 定时器1中断服务程序
{
static unsigned int cnt = 0;
TH1 = 0xFD;
TL1 = 0xFD;
cnt++;
if(cnt >= 1000) // 5s
{
cnt = 0;
LED = 0; // 熄灭 LED
}
}
```
以下是 STM32 的代码:
```c
#include "stm32f10x.h"
#define LED_PIN GPIO_Pin_13 // LED 引脚号
#define LED_PORT GPIOC // LED 端口号
void delay_ms(unsigned int n) // 延时函数
{
while(n--)
{
unsigned int i = 1000;
while(i--);
}
}
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE); // 使能 GPIOC 时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE); // 使能 USART1 时钟
// 配置 LED 引脚为推挽输出
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
// 配置 USART1 引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; // USART1_TX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; // USART1_RX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置 USART1 参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE); // 使能 USART1
while(1)
{
if(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == SET) // 检测是否接收到数据
{
char ch = USART_ReceiveData(USART1);
if(ch == '1') // 如果接收到 '1'
{
GPIO_SetBits(LED_PORT, LED_PIN); // 点亮 LED
delay_ms(5000); // 延时 5s
GPIO_ResetBits(LED_PORT, LED_PIN); // 熄灭 LED
}
}
}
}
```
阅读全文
相关推荐
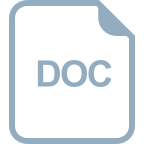
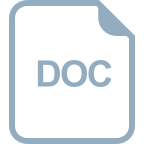
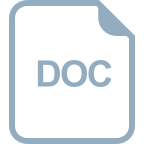

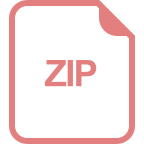
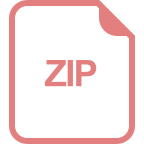
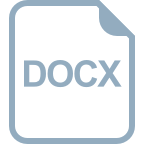
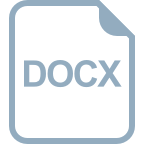
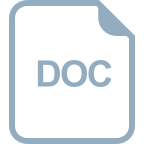
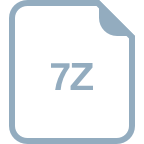
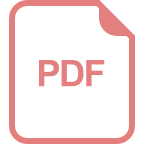
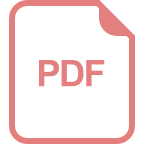
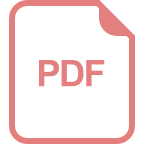
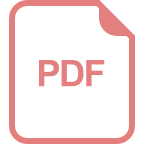
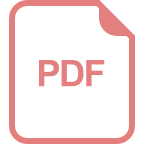
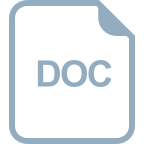
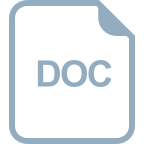
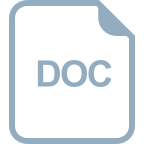
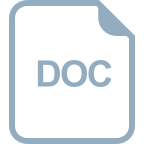