遗传算法python三维
时间: 2023-09-03 12:07:19 浏览: 158
遗传算法是一种模拟自然界进化过程的优化算法,它可以用于解决各种问题,包括三维问题。在Python中,你可以使用遗传算法库来实现三维问题的优化。
首先,你需要定义问题的适应度函数,用于评估每个个体的优劣程度。对于三维问题,适应度函数可以是目标函数的值,比如最小化或最大化某个函数。
接下来,你需要定义遗传算法的基本组成部分:选择、交叉和变异。选择过程通过评估个体的适应度来选择优秀的个体作为下一代的父代。交叉过程通过交换两个个体的染色体片段来产生新的个体。变异过程通过随机改变某个个体的染色体中的基因来引入新的遗传信息。
最后,你需要初始化一组初始个体,并迭代进行选择、交叉和变异操作,优化得到最优解。
下面是一个简单的示例代码,演示了如何使用遗传算法库`deap`来解决一个简单的三维问题:
```python
import random
from deap import base, creator, tools
# 定义问题的适应度函数
def evaluate(individual):
# 这里假设三维问题是最小化x^2 + y^2 + z^2
x, y, z = individual
return x**2 + y**2 + z**2,
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
# 定义个体的基因生成方式
toolbox.register("attribute", random.uniform, -10, 10)
# 定义个体生成方式
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attribute, n=3)
# 定义种群生成方式
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 定义遗传算法的评价函数、交叉操作和变异操作
toolbox.register("evaluate", evaluate)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=1, indpb=0.1)
toolbox.register("select", tools.selTournament, tournsize=3)
def main():
population = toolbox.population(n=50)
CXPB, MUTPB, NGEN = 0.5, 0.2, 20
for gen in range(NGEN):
offspring = algorithms.varAnd(population, toolbox, cxpb=CXPB, mutpb=MUTPB)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
population = toolbox.select(offspring, k=len(population))
best_individual = tools.selBest(population, k=1)[0]
print("Best individual:", best_individual)
print("Fitness:", best_individual.fitness.values[0])
if __name__ == "__main__":
main()
```
这只是一个简单的示例,你可以根据具体的问题进行适当的修改。希望对你有帮助!
阅读全文
相关推荐

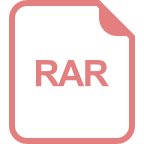
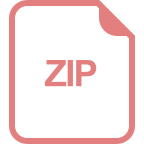
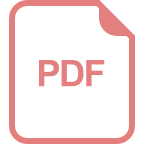
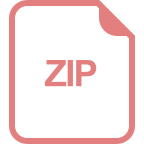
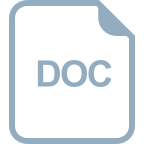
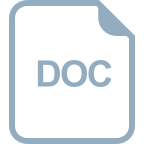
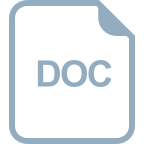



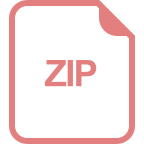
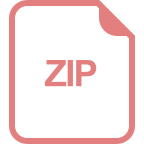
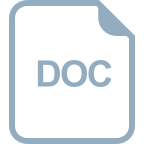
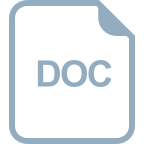
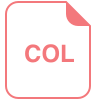