Python遗传算法框架搭建:从零开始的必经之路
发布时间: 2024-08-31 17:10:08 阅读量: 56 订阅数: 24 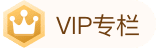
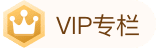
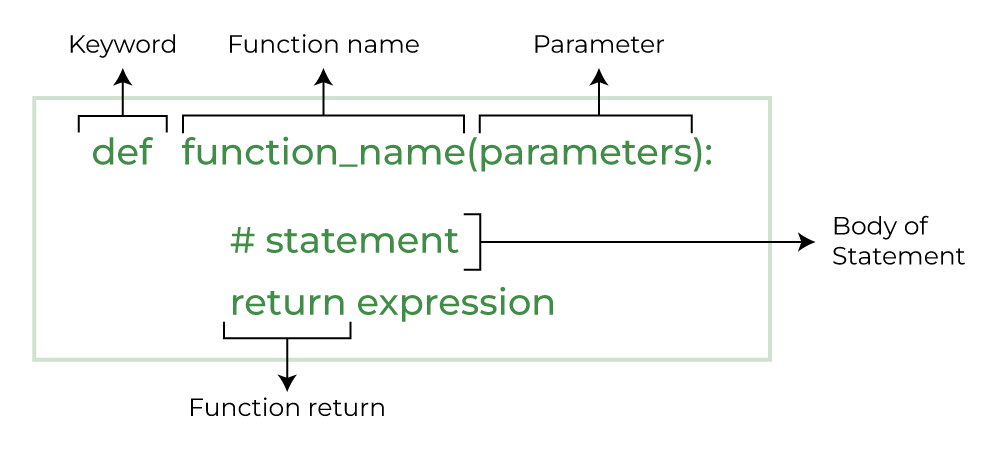
# 1. 遗传算法的基本原理与概念
遗传算法是一种模拟生物进化过程的优化算法,它通过自然选择、遗传和变异等生物进化机制来寻找最优解。本章旨在介绍遗传算法的基本原理和核心概念,为读者提供一个全面的基础知识体系。
## 1.1 遗传算法的历史与发展
遗传算法(Genetic Algorithms,GAs)由John Holland教授于1975年首次提出,其灵感来源于达尔文的自然选择理论。Holland发现,通过模拟自然选择和遗传机制可以有效解决优化问题。随后,遗传算法在理论和应用领域都得到了广泛的发展和研究。
## 1.2 遗传算法的工作原理
遗传算法通过模拟自然遗传过程在潜在解集合中进行搜索。它通常涉及以下步骤:初始化一个种群,评估每个个体的适应度,选择优秀的个体进行交叉与变异,生成新的种群,重复以上步骤直至达到终止条件。
## 1.3 遗传算法的基本组成
遗传算法主要由三个基本操作组成:选择(Selection),交叉(Crossover)和变异(Mutation)。选择操作模拟自然选择,允许适应度高的个体更多地繁殖后代;交叉操作模仿生物的染色体交换,产生新的个体;变异操作则引入随机性,以维持种群的多样性。
```mermaid
flowchart LR
A[初始化种群] --> B[评估适应度]
B --> C[选择优秀个体]
C --> D[交叉与变异产生新种群]
D --> E[新种群评估适应度]
E --> |满足条件| F[输出最优解]
E --> |不满足条件| C
```
以上流程图简明地概括了遗传算法的基本工作流程,接下来的章节将深入探讨遗传算法的各个组件及其在Python中的实现方式。
# 2. Python遗传算法框架搭建理论基础
## 2.1 遗传算法核心组件解析
### 2.1.1 个体与种群的表示
在遗传算法中,个体通常是指解决方案的一个实例,而种群则是由多个个体组成的集合。在Python中,我们可以使用列表或数组来表示一个个体,而种群则是一个包含多个个体的列表或数组。
个体可以使用Python字典或对象来表示,其中键或属性是变量,值是这些变量的解。
```python
# 示例:个体表示
individual = {
"x": 5,
"y": 10
}
```
种群表示可以是一个包含个体的列表:
```python
# 示例:种群表示
population = [
{"x": 5, "y": 10},
{"x": 7, "y": 3},
# ...
]
```
在设计遗传算法时,个体和种群的设计至关重要。个体的设计要能够准确表示问题的解,而种群的大小则影响算法的搜索能力及收敛速度。
### 2.1.2 适应度函数的设计
适应度函数用于评估每个个体的适应程度,它的重要性等同于自然选择中的“适者生存”原则。在遗传算法中,高适应度的个体有更高的机会被选中用于繁殖下一代。
适应度函数的设计取决于具体问题。以最大化问题为例,我们可以将适应度函数设计为解的目标函数值。而最小化问题则需要将其转换为最大化问题,通常通过取目标函数值的负值或倒数。
```python
# 示例:适应度函数设计
def fitness_function(individual):
# 假设我们要解决的是最大化问题
# 此处以一个简单的数学表达式为例
return individual['x'] + individual['y']
```
适应度函数的选择直接关系到算法的效率和收敛性。复杂问题可能需要复杂的设计,例如结合惩罚项来处理约束条件。
## 2.2 选择机制的实现
选择机制负责从当前种群中选择个体用于产生下一代。选择机制的核心思想是让适应度高的个体有更大的机会被选中。
### 2.2.1 轮盘赌选择
轮盘赌选择(Roulette Wheel Selection)是一种广泛使用的选择机制,它基于个体适应度与种群总适应度的比例来确定被选中概率。
```python
import random
def roulette_wheel_selection(population, fitness_scores):
# 计算总适应度
total_fitness = sum(fitness_scores)
# 生成一个在[0, total_fitness)区间内的随机数
pick = random.uniform(0, total_fitness)
current = 0
for individual, score in zip(population, fitness_scores):
current += score
if current > pick:
return individual
return individual
```
轮盘赌选择模拟了“机会主义”的选择方式,虽然简单,但存在较高适应度个体“垄断”选择的可能。
### 2.2.2 锦标赛选择
锦标赛选择(Tournament Selection)方法是通过随机选择若干个体,然后在这些个体中选择适应度最高的一个。
```python
import random
def tournament_selection(population, fitness_scores, tournament_size=2):
selected = random.sample(population, tournament_size)
selected_fitness = [fitness_scores[population.index(ind)] for ind in selected]
winner = selected[selected_fitness.index(max(selected_fitness))]
return winner
```
锦标赛选择避免了轮盘赌选择中的高适应度个体“垄断”问题,同时实现起来也较为简单。
### 2.2.3 随机选择及其他变种
随机选择机制指完全随机地从种群中选取个体。这通常作为基准用于与其他选择机制进行比较。
```python
def random_selection(population):
return random.choice(population)
```
遗传算法中还有许多其他选择机制,如稳态选择、排名选择等,每种机制都有其适用场景和优势。
## 2.3 交叉与变异操作策略
交叉与变异是遗传算法中模拟生物遗传过程的关键操作。交叉负责根据两个父代个体产生子代,而变异则引入新的遗传多样性。
### 2.3.1 交叉点的选择与实现
交叉点的选择对算法性能有显著影响。单点交叉是交叉操作中最简单的一种,它在某个随机点将两个父代个体的基因串切开并交换。
```python
def single_point_crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1)-1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
```
双点交叉和多点交叉是单点交叉的扩展。选择不同的交叉点数量和位置会对算法结果产生影响。
### 2.3.2 变异概率与类型
变异操作通过随机改变个体的某些基因来引入新的遗传多样性。变异概率是决定变异发生的频率。
```python
def mutate(individual, mutation_rate):
mutated_individual = individual.copy()
for gene in mutated_individual:
if random.random() < mutation_rate:
mutated_individual[gene] = random.choice(range(gene_possible_values[gene]))
return mutated_individual
```
变异类型可以是简单的基因值变动,也可以是更复杂的操作,如基因重排或逆转变异。
### 2.3.3 高级交叉与变异技术
高级交叉和变异技术在遗传算法中用于解决特定问题。例如,均匀交叉(Uniform Crossover)在每个基因位上随机决定父代基因的来源。
```python
def uniform_crossover(parent1, parent2):
child = {}
for gene in parent1:
child[gene] = random.choice([parent1[gene], parent2[gene]])
return child
```
高级变异技术如多点变异、高斯变异等,能够使算法更加灵活和高效。
为了能够更直观地展示上述章节内容,接下来通过一个表格和流程图对选择机制的实现细节进行总结。
| 选择机制名称 | 描述 | 实现方法 | 特点 |
|-------------|------|----------|------|
| 轮盘赌选择 | 基于个体适应度与总适应度比例进行选择 | 计算适应度总和,按比例选择个体 | 适合平衡选择压力和多样性 |
| 锦标赛选择 | 在随机选取的若干个体中选择最佳者 | 从种群随机选取若干个体,选取其中适应度最高的个体 | 保留优秀个体的同时引入多样性 |
| 随机选择 | 完全随机选择个体 | 随机从种群中选取个体 | 保证了种群多样性,但可能会选择到适应度较低的个体 |
接下来,我们使用mermaid流程图来描述轮盘赌选择的逻辑:
```mermaid
flowchart TD
A[开始] --> B[计算总适应度]
B --> C[随机选择指针]
C --> D{比较指针与适应度}
D -- 如果 > -- > E[选择当前个体]
D -- 如果 < -- > C
E --> F[返回选择的个体]
F --> G[结束]
```
通过以上表格和流程图,我们可以清晰地看到不同选择机制的设计和逻辑差异,有助于在实际应用中选择合适的策略。
# 3. 基于Python的遗传算法框架实践
## 3.1
0
0
相关推荐
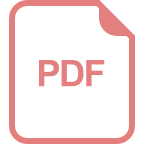
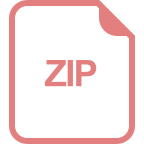





