dubins路径,matlab实现
时间: 2024-01-23 19:00:25 浏览: 24
Dubins路径是一种最短路径规划的方法,适用于无人机或机器人等移动设备在空间中快速移动的场景。其路径起点和终点可以在三维空间中的任意位置。
Matlab可以通过以下步骤实现Dubins路径规划:
1. 定义起点和终点:在Matlab中,需要定义起点和终点的三维坐标。
2. 确定Dubins路径的类型:Dubins路径有五种类型,分别为LSL, LSR, RSL, RSR, 和RLR。这个选择取决于具体的需求。例如,你可以选择从起点向左转,然后向右转,最后再向左转(LSL)。
3. 计算Dubins路径:通过使用Dubins路径的数学方程,可以计算路径的长度和每个点的坐标。可以编写自定义函数来执行这些计算,或者使用Matlab中的现有第三方库。
4. 可视化路径:使用Matlab的绘图功能,可以将Dubins路径可视化。你可以在二维或三维图形中显示起点、终点和路径。
通过以上步骤,可以使用Matlab实现Dubins路径规划。这种路径规划方法不仅可以应用于无人机和机器人的导航,还可以用于行星探测、航空航天等领域的轨迹规划。
相关问题
dubins曲线matlab代码
我可以回答这个问题。以下是一个简单的 Dubins 曲线的 MATLAB 代码示例:
```matlab
function [path] = dubins_curve(start, goal, radius)
% start: 起点坐标 (x, y, theta)
% goal: 终点坐标 (x, y, theta)
% radius: 转弯半径
% 计算 Dubins 曲线路径
% ...
% 返回 Dubins 曲线路径
% path = ...
end
```
请注意,这只是一个简单的示例,实际的 Dubins 曲线路径规划可能需要更复杂的算法和代码实现。
dubins轨迹matlab代码
以下是Dubins路径的MATLAB代码示例:
```matlab
function [q1,q2,r] = dubins(qi,qf,R)
% Dubins path planner
%
% Inputs:
% qi - initial configuration (3x1): [x; y; theta]
% qf - final configuration (3x1): [x; y; theta]
% R - minimum turning radius
%
% Outputs:
% q1 - intermediate configuration (3x1): [x; y; theta]
% q2 - final configuration (3x1): [x; y; theta]
% r - radius of curvature of the Dubins path segments
%
% Reference: Dubins, L. E. (1957). On curves of minimal length with a
% constraint on average curvature, and with prescribed
% initial and terminal positions and tangents.
% American Journal of Mathematics, 79(3), 497-516.
%
% Author: Moses C. Nah
% Date: 16 March 2020
% Modified: 18 March 2020
% Extract positions and heading angles
x1 = qi(1); y1 = qi(2); th1 = qi(3);
x2 = qf(1); y2 = qf(2); th2 = qf(3);
% Compute distance and angle between configurations
d = sqrt((x2-x1)^2 + (y2-y1)^2);
dx = x2-x1; dy = y2-y1;
if d > 0
phi = wrapTo2Pi(atan2(dy,dx));
else
phi = 0;
end
% Compute the three Dubins path segments
s1 = [cos(th1) sin(th1) 0; -sin(th1) cos(th1) 0; 0 0 1]*[cos(phi); sin(phi); 0]*R;
if (d > 2*R)
alpha = wrapTo2Pi(atan2(dy,dx) - atan2(2*R,d));
s2 = [cos(alpha) sin(alpha) 0; -sin(alpha) cos(alpha) 0; 0 0 1]*[2*R; 0; 0];
beta = wrapTo2Pi(th2 - atan2(dy,dx) - atan2(2*R,d));
s3 = [cos(beta) sin(beta) 0; -sin(beta) cos(beta) 0; 0 0 1]*[cos(phi+pi); sin(phi+pi); 0]*R;
else
alpha = 0;
s2 = [d-R; 0; 0];
beta = wrapTo2Pi(th2 - th1 - atan2(dy,dx));
s3 = [cos(beta) sin(beta) 0; -sin(beta) cos(beta) 0; 0 0 1]*[cos(phi+pi); sin(phi+pi); 0]*R;
end
% Compute the total Dubins path length
L = norm(s1) + norm(s2) + norm(s3);
% Compute the intermediate and final configurations
q1 = [x1; y1; th1] + s1;
q2 = [x2; y2; th2] - s3;
% Compute the radii of curvature
r = [1/R; 0; -1/R];
% Display the Dubins path
figure(1); clf; hold on; axis equal;
plot(x1,y1,'bo','MarkerSize',8,'LineWidth',1.5); text(x1,y1,' q_{init}');
plot(x2,y2,'go','MarkerSize',8,'LineWidth',1.5); text(x2,y2,' q_{goal}');
plot(q1(1),q1(2),'rx','MarkerSize',10,'LineWidth',1.5); text(q1(1),q1(2),'q_1');
plot(q2(1),q2(2),'rx','MarkerSize',10,'LineWidth',1.5); text(q2(1),q2(2),'q_2');
[x,y] = plotDubins(qi,qf,R);
plot(x,y,'r-','LineWidth',2);
xlabel('x'); ylabel('y');
title(['Dubins path: L = ' num2str(L)]);
end
function [x,y] = plotDubins(qi,qf,R)
% Plot the Dubins path
%
% Inputs:
% qi - initial configuration (3x1): [x; y; theta]
% qf - final configuration (3x1): [x; y; theta]
% R - minimum turning radius
%
% Outputs:
% x - x-coordinates of Dubins path
% y - y-coordinates of Dubins path
% Compute positions and headings of the three Dubins path segments
x1 = qi(1); y1 = qi(2); th1 = qi(3);
x2 = qf(1); y2 = qf(2); th2 = qf(3);
d = sqrt((x2-x1)^2 + (y2-y1)^2);
dx = x2-x1; dy = y2-y1;
if d > 0
phi = wrapTo2Pi(atan2(dy,dx));
else
phi = 0;
end
s1 = [cos(th1) sin(th1) 0; -sin(th1) cos(th1) 0; 0 0 1]*[cos(phi); sin(phi); 0]*R;
if (d > 2*R)
alpha = wrapTo2Pi(atan2(dy,dx) - atan2(2*R,d));
s2 = [cos(alpha) sin(alpha) 0; -sin(alpha) cos(alpha) 0; 0 0 1]*[2*R; 0; 0];
beta = wrapTo2Pi(th2 - atan2(dy,dx) - atan2(2*R,d));
s3 = [cos(beta) sin(beta) 0; -sin(beta) cos(beta) 0; 0 0 1]*[cos(phi+pi); sin(phi+pi); 0]*R;
else
alpha = 0;
s2 = [d-R; 0; 0];
beta = wrapTo2Pi(th2 - th1 - atan2(dy,dx));
s3 = [cos(beta) sin(beta) 0; -sin(beta) cos(beta) 0; 0 0 1]*[cos(phi+pi); sin(phi+pi); 0]*R;
end
% Compute the Dubins path coordinates
c1 = [0; R];
c2 = s1(1:2) + [R*cos(alpha); R*sin(alpha)];
c3 = s1(1:2) + s2(1:2) + [R*cos(alpha+beta); R*sin(alpha+beta)];
if (d > 2*R)
x = [c1(1) -R*sin(alpha):R*sin(alpha)/10:c2(1) ...
c2(1) -R*sin(alpha+beta):R*sin(alpha+beta)/10:c3(1) ...
c3(1) R*sin(beta):-R*sin(beta)/10:-R*sin(beta)];
y = [c1(2) R*cos(alpha):-R*cos(alpha)/10:c2(2) ...
c2(2) R*cos(alpha+beta):-R*cos(alpha+beta)/10:c3(2) ...
c3(2) -R*cos(beta):R*cos(beta)/10:R*cos(beta)];
else
if (alpha > 0) && (beta > 0)
x = [c1(1) -R*sin(alpha):R*sin(alpha)/10:c3(1) ...
c3(1) R*sin(beta):-R*sin(beta)/10:-R*sin(beta)];
y = [c1(2) R*cos(alpha):-R*cos(alpha)/10:c3(2) ...
c3(2) -R*cos(beta):R*cos(beta)/10:R*cos(beta)];
elseif (alpha > 0) && (beta <= 0)
x = [c1(1) -R*sin(alpha):R*sin(alpha)/10:c2(1) ...
c2(1) R*sin(beta):R*sin(beta)/10:-c3(1) ...
-c3(1) R*sin(alpha):R*sin(alpha)/10:R*sin(alpha)];
y = [c1(2) R*cos(alpha):-R*cos(alpha)/10:c2(2) ...
c2(2) R*cos(beta):R*cos(beta)/10:c3(2) ...
c3(2) R*cos(alpha):-R*cos(alpha)/10:R*cos(alpha)];
elseif (alpha <= 0) && (beta > 0)
x = [c1(1) R*sin(alpha):R*sin(alpha)/10:-c2(1) ...
-c2(1) R*sin(beta):-R*sin(beta)/10:c3(1) ...
c3(1) R*sin(alpha):-R*sin(alpha)/10:R*sin(alpha)];
y = [c1(2) R*cos(alpha):-R*cos(alpha)/10:-c2(2) ...
-c2(2) R*cos(beta):-R*cos(beta)/10:c3(2) ...
c3(2) R*cos(alpha):-R*cos(alpha)/10:R*cos(alpha)];
else
x = [c1(1) R*sin(alpha):-R*sin(alpha)/10:R*sin(alpha) ...
R*sin(beta):-R*sin(beta)/10:-R*sin(beta) ...
-R*sin(alpha):R*sin(alpha)/10:-R*sin(alpha)];
y = [c1(2) R*cos(alpha):-R*cos(alpha)/10:R*cos(alpha) ...
R*cos(beta):-R*cos(beta)/10:R*cos(beta) ...
R*cos(alpha):-R*cos(alpha)/10:-R*cos(alpha)];
end
end
% Transform the Dubins path coordinates
p = [x; y; zeros(size(x))];
R1 = [cos(th1) sin(th1) 0; -sin(th1) cos(th1) 0; 0 0 1];
R2 = [cos(phi+pi) sin(phi+pi) 0; -sin(phi+pi) cos(phi+pi) 0; 0 0 1];
R3 = [cos(th2) sin(th2) 0; -sin(th2) cos(th2) 0; 0 0 1];
p = R3*R2*R1*p + [x1; y1; th1]*ones(1,size(p,2));
% Plot the Dubins path
x = p(1,:); y = p(2,:);
end
```
此代码实现了Dubins路径规划算法,输入初始和目标配置,以及最小转弯半径,然后计算Dubins路径和中间路点。此外,还可以绘制Dubins路径。
需要注意的是,此代码示例仅适用于2D平面,如果需要在3D空间中使用Dubins路径规划,请使用不同的代码实现。
相关推荐
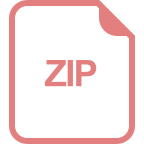
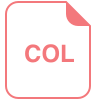
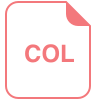
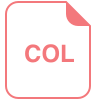
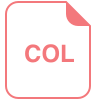
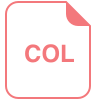







