检查一下我的这个函数max_pairwise_distance
时间: 2024-08-14 20:08:38 浏览: 41
您提供的`max_pairwise_distance`函数看起来基本合理,它的核心逻辑是:
1. 计算所有点对间的距离,存入`distances`列表。
2. 对`distances`列表使用冒泡排序算法进行排序。
3. 返回排序后的列表中的最后一个元素,即最大距离。
然而,这个函数假设了传入的`X`是一个包含坐标点的二维列表,每个点表示为一对 `[x, y]`。如果没有进行有效性检查(例如,确认输入是否是正确的列表结构,或者确保有至少两个点),在实际应用中可能会遇到问题。
另外,使用冒泡排序虽然简单直观,但对于大数据量的情况效率较低。对于较大的数据集,可以考虑使用更高效的排序算法,如快速排序、归并排序或者内置的`sorted`函数配合`lambda`表达式。
为了更好地帮助您,如果您能提供一个具体的`X`实例,我可以帮您运行这个函数并演示其效果。同时,如果您需要改进性能或添加错误处理,我们可以讨论相应的优化策略。
相关问题
请解释一下 def forward(self, input_text, positive_text, negative_text): distance_positive = F.pairwise_distance(input_text, positive_text) distance_negative = F.pairwise_distance(input_text, negative_text) loss = torch.mean((distance_positive - distance_negative + self.margin).clamp(min=0)) return loss
这段代码实现了一个三元组损失函数,在训练过程中用于学习如何将输入文本与正样本文本更接近,而与负样本文本更远离。其中,输入文本、正样本文本和负样本文本都被表示为向量形式,且经过了相同的文本编码器得到。
具体来说,这段代码的功能如下:
1. 通过调用 PyTorch 中的 F.pairwise_distance 函数计算输入文本与正样本文本、输入文本与负样本文本之间的欧氏距离,得到 distance_positive 和 distance_negative。
2. 根据三元组损失函数的公式,计算损失值 loss。公式为:
loss = max(distance_positive - distance_negative + margin, 0)
其中,margin 是一个预先设定的常数,用于控制输入文本与正样本文本之间的距离与输入文本与负样本文本之间的距离之间的差异。如果两者之间的差异小于 margin,则损失值为 0;否则,损失值为两者之间的差异。
3. 最后,将损失值返回。在训练过程中,该损失函数会被作为模型的目标函数,通过反向传播来更新模型的参数,以使模型能够更好地区分输入文本和正负样本文本之间的差异。
python自定义损失函数
在Python中,可以通过几种方式自定义损失函数。其中一种方法是直接使用PyTorch中的函数接口和Python内建的方法来定义。在这种方法中,你可以定义一个继承nn.Module的类,重写该类的__init__()和forward()方法。在__init__()方法中,你可以初始化超参数;在forward()方法中,你可以定义损失的计算方式,并进行前向传播。例如,你可以使用torch.mean()函数计算损失的均值,使用torch.pow()函数计算预测值和标签之间的差的平方。你也可以使用torch.clamp()函数来对损失进行裁剪。最后,返回计算得到的损失值。以下是一个示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as func
class MyLoss(nn.Module):
def __init__(self, param1):
super(MyLoss, self).__init__()
self.param1 = param1
def forward(self, predict, label):
loss = torch.mean(torch.pow((predict - label), 2))
return loss
```
另一种方法是扩展PyTorch库中已有的损失函数类。你可以定义一个继承nn.Module的类,并重写该类的__init__()和forward()方法。在__init__()方法中,你可以初始化超参数;在forward()方法中,你可以根据自定义的计算方式来计算损失,并返回损失值。这种方法可以方便地使用PyTorch提供的数学函数,如torch.clamp()和torch.pairwise_distance()。以下是一个示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as func
class TripletLossFunc(nn.Module):
def __init__(self, t1, t2, beta):
super(TripletLossFunc, self).__init__()
self.t1 = t1
self.t2 = t2
self.beta = beta
def forward(self, anchor, positive, negative):
matched = torch.pow(func.pairwise_distance(anchor, positive), 2)
mismatched = torch.pow(func.pairwise_distance(anchor, negative), 2)
part_1 = torch.clamp(matched - mismatched, min=self.t1)
part_2 = torch.clamp(matched, min=self.t2)
dist_hinge = part_1 + self.beta * part_2
loss = torch.mean(dist_hinge)
return loss
```
这些方法都可以在自定义损失函数时使用,具体选择哪种方法取决于你的需求和个人偏好。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [PyTorch自定义损失函数](https://blog.csdn.net/weixin_43424482/article/details/118709610)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [pytorch基础使用—自定义损失函数](https://blog.csdn.net/weixin_51691064/article/details/130138001)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
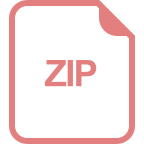
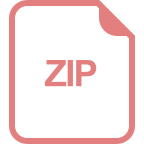
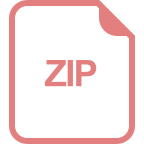
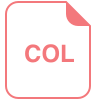
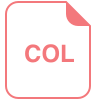
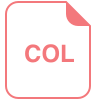
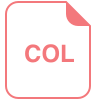
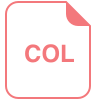





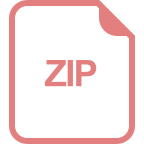
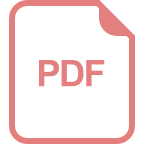