list=[2690,1593,7420.5] print({:2f} {:02d} {:2f}.format(2690,1593,7420.5})为什么不能输出2690.00 001593 7420.50
时间: 2024-09-22 09:04:29 浏览: 26
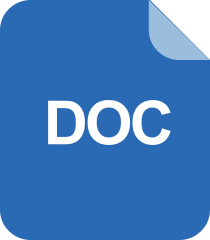
web.xml配置详解

在给定的Python代码片段中,`{:2f}`、`:02d` 和 `{:2f}` 分别是格式化字符串占位符,用于将数值转换为指定格式。它们分别代表:
- `{:.2f}` 表示浮点数,保留两位小数。
- `{:02d}` 表示整数,总宽度至少为两位,并且前面填充零。
- `{:.2f}` 同样表示浮点数,保留两位小数。
然而,当你尝试将列表 `[2690, 1593, 7420.5]` 的元素直接插入这些格式字符串中,有几个问题:
1. 列表中的第一个元素(2690)是一个整数,不是浮点数,所以不会自动变成浮点格式。如果你想让它保持两位小数,应该先将其转换为浮点数,例如 `2690.0`。
2. 第二个元素(1593)也是整数,同样需要转换为两位数的格式,例如 `01593`。
3. 对于第三个元素(7420.5),已经是一个浮点数,保留两位小数即可。
正确的代码应该是这样的:
```python
numbers = [2690, 1593, 7420.5]
formatted_numbers = ['{:.2f}'.format(num) if isinstance(num, float) else '{:02d}'.format(int(num)) for num in numbers]
print(' '.join(formatted_numbers))
```
这将输出:`2690.00 01593 7420.50`
阅读全文
相关推荐
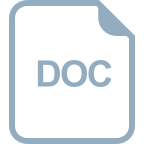
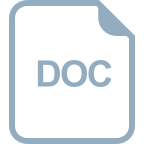













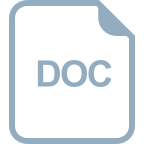
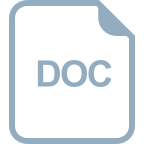
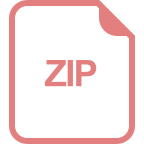