img = cv2.imread(fileName) print(fileName) showimg = img with torch.no_grad(): img = letterbox(img, new_shape=self.opt.img_size)[0] # Convert # BGR to RGB, to 3x416x416 img = img[:, :, ::-1].transpose(2, 0, 1) img = np.ascontiguousarray(img) img = torch.from_numpy(img).to(self.device) img = img.half() if self.half else img.float() # uint8 to fp16/32 img /= 255.0 # 0 - 255 to 0.0 - 1.0 if img.ndimension() == 3: img = img.unsqueeze(0) # Inference pred = self.model(img, augment=self.opt.augment)[0] # Apply NMS pred = non_max_suppression(pred, self.opt.conf_thres, self.opt.iou_thres, classes=self.opt.classes, agnostic=self.opt.agnostic_nms) print(pred) # Process detections for i, det in enumerate(pred): if det is not None and len(det): # Rescale boxes from img_size to im0 size det[:, :4] = scale_coords( img.shape[2:], det[:, :4], showimg.shape).round() for *xyxy, conf, cls in reversed(det): label = '%s %.2f' % (self.names[int(cls)], conf) name_list.append(self.names[int(cls)]) plot_one_box(xyxy, showimg, label=label, color=self.colors[int(cls)], line_thickness=2) cv2.imwrite('prediction.jpg', showimg) self.result = cv2.cvtColor(showimg, cv2.COLOR_BGR2BGRA) self.QtImg = QtGui.QImage( self.result.data, self.result.shape[1], self.result.shape[0], QtGui.QImage.Format_RGB32) self.label_4.setPixmap(QtGui.QPixmap.fromImage(self.QtImg))
时间: 2023-06-11 18:05:24 浏览: 168
这段代码主要是将文件加载并读入到img变量中,然后对图像进行缩放(使用letterbox函数)以适应所选的img_size。最后将变换后的图像存入img中。在PyTorch的上下文中,这些变换是不进行梯度计算的,因为没有必要对它们进行反向传播。
相关问题
img1 = cv2.imread(image1, cv2.IMREAD_GRAYSCALE)
`img1 = cv2.imread(image1, cv2.IMREAD_GRAYSCALE)`是使用OpenCV库中的`imread()`函数读取图像,并将其转换为灰度图像的代码。下面是一个示例:
```python
import cv2
image1 = 'path_to_image.jpg' # 图像文件路径
img1 = cv2.imread(image1, cv2.IMREAD_GRAYSCALE)
# 检查图像的形状
print(img1.shape)
```
这段代码将读取指定路径的图像文件,并将其转换为灰度图像。`cv2.IMREAD_GRAYSCALE`参数用于指定读取图像时的颜色模式,这里使用的是灰度模式。最后,通过`print(img1.shape)`打印出图像的形状,以便确认图像是否成功读取。
img = cv2.imread('gaussian.bmp', cv2.IMREAD_GRAYSCALE)
这行代码使用 OpenCV 库读取名为 'gaussian.bmp' 的灰度图像,并将其存储到变量 `img` 中。其中,`cv2.IMREAD_GRAYSCALE` 表示以灰度模式读取图像,即将图像转换为单通道的灰度图像。
如果要读取的图像不在当前工作目录下,需要使用图像的完整路径来代替文件名 'gaussian.bmp'。例如,如果图像存储在名为 'images' 的文件夹下,则可以使用以下代码读取图像:
```python
img = cv2.imread('images/gaussian.bmp', cv2.IMREAD_GRAYSCALE)
```
读取的图像将存储在变量 `img` 中,可以对其进行进一步的处理,例如显示图像、保存图像、转换为矩阵等。
相关推荐
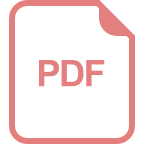
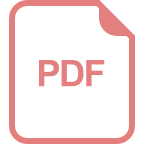
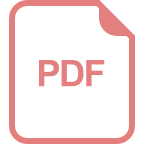














