java。oop 写测试三角形形状
时间: 2024-09-20 20:15:23 浏览: 68
在 Java 中,OOP (Object-Oriented Programming) 提供了一种结构化的编程方式,它强调封装、继承和多态等核心原则。如果你想要编写一个测试三角形形状的程序,首先你需要创建一个表示三角形的类,这个类应该包含三角形的基本属性如边长,并实现必要的方法,比如计算面积。
```java
// 三角形类
public class Triangle {
private double side1;
private double side2;
private double side3;
// 构造函数
public Triangle(double a, double b, double c) {
if (a + b > c && a + c > b && b + c > a) {
this.side1 = a;
this.side2 = b;
this.side3 = c;
} else {
throw new IllegalArgumentException("Invalid triangle sides");
}
}
// 计算面积的方法,使用海伦公式
public double getArea() {
double s = (side1 + side2 + side3) / 2; // 半周长
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
}
```
然后你可以创建一个单元测试类,使用 JUnit 或者 TestNG 等测试框架来验证三角形的行为:
```java
import org.junit.Test;
import static org.junit.Assert.*;
public class TriangleTest {
@Test
public void testTriangleArea() {
Triangle triangle = new Triangle(3, 4, 5); // 正三角形
double expectedArea = 6.0;
assertEquals(expectedArea, triangle.getArea(), 0.001);
triangle = new Triangle(2, 3, 4); // 直角三角形
expectedArea = 3.0;
assertEquals(expectedArea, triangle.getArea(), 0.001);
try {
new Triangle(1, 1, 3); // 无法构成三角形
fail("Expected an exception to be thrown.");
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage()); // 输出 "Invalid triangle sides"
}
}
}
```
在这个例子中,我们测试了正三角形和直角三角形的面积,同时也处理了无效的三角形边长输入情况。
阅读全文
相关推荐
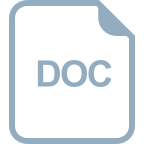
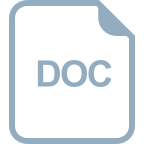
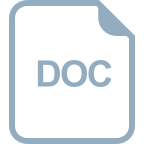

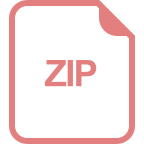
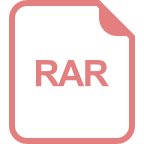
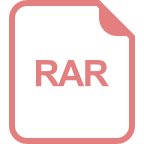
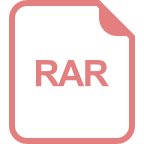
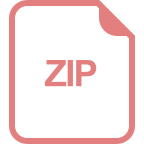
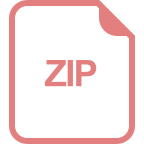
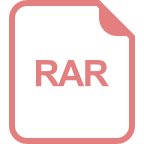
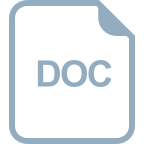
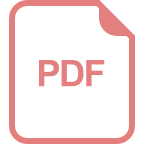
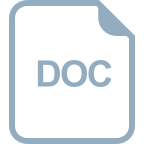
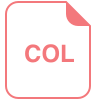
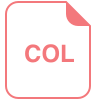
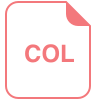
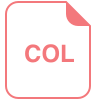
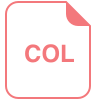