spring boot 读取指定路径的文件,返回文件流
时间: 2023-12-27 18:03:36 浏览: 36
在Spring Boot中,你可以使用`Resource`和`InputStream`来读取指定路径的文件,并将文件内容返回为文件流。以下是一个示例代码:
```java
import org.springframework.core.io.FileSystemResource;
import org.springframework.core.io.Resource;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class FileController {
@GetMapping("/file/{filename}")
public ResponseEntity<Resource> getFile(@PathVariable String filename) throws IOException {
// 指定文件路径
String filePath = "/path/to/your/file/" + filename;
// 创建文件资源对象
Resource resource = new FileSystemResource(filePath);
// 检查文件是否存在
if (!resource.exists()) {
return ResponseEntity.notFound().build();
}
// 获取文件输入流
InputStream inputStream = resource.getInputStream();
// 返回文件流
return ResponseEntity.ok()
.contentLength(Files.size(Paths.get(filePath)))
.header("Content-Disposition", "attachment; filename=\"" + filename + "\"")
.body(new InputStreamResource(inputStream));
}
}
```
在上述代码中,你需要将`/path/to/your/file/`替换为实际存储文件的路径。当访问`/file/{filename}`时,该方法会检查文件是否存在,然后将文件内容返回为文件流。
请注意,上述示例假设文件路径是绝对路径。如果你需要处理相对路径或基于类路径的文件,请使用适当的方法获取资源。
希望这对你有所帮助!如果你有任何其他问题,请随时提问。
相关推荐
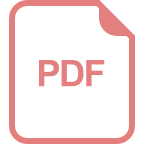
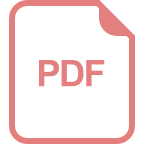














