spring boot 读取服务器文件,返回Blob
时间: 2024-01-29 12:04:32 浏览: 228
要在Spring Boot中读取服务器上的文件并返回Blob对象,你可以使用`Resource`和`InputStream`来读取文件内容,并使用`ByteArrayOutputStream`将内容转换为字节数组,最后通过`Blob`类型返回。以下是一个示例代码:
```java
import org.springframework.core.io.Resource;
import org.springframework.core.io.InputStreamResource;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Blob;
import java.sql.SQLException;
@RestController
public class FileController {
@GetMapping("/file/{filename}")
public ResponseEntity<Resource> getFile(@PathVariable String filename) throws IOException, SQLException {
// 读取服务器上的文件
InputStream inputStream = getClass().getResourceAsStream("/path/to/your/file/" + filename);
// 将文件内容转换为字节数组
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
byte[] fileBytes = outputStream.toByteArray();
// 创建Blob对象
final Blob blob = new javax.sql.rowset.serial.SerialBlob(fileBytes);
// 返回Blob对象
return ResponseEntity.ok()
.contentLength(blob.length())
.header("Content-Disposition", "attachment; filename=\"" + filename + "\"")
.body(new InputStreamResource(blob.getBinaryStream()));
}
}
```
在上面的代码中,你需要将`/path/to/your/file/`替换为实际存储文件的路径。当访问`/file/{filename}`时,该方法会读取文件内容并返回一个带有Blob对象的ResponseEntity。此外,你还可以根据需要调整代码以适应你的应用需求。
请注意,上述示例假设文件位于类路径下,可以通过`getClass().getResourceAsStream()`来获取文件的输入流。如果文件位于文件系统中的特定路径下,你可以使用`FileInputStream`来读取文件。
希望这对你有所帮助!如果你有任何其他问题,请随时提问。
阅读全文
相关推荐
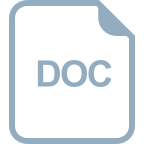
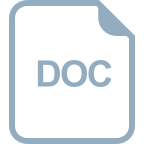
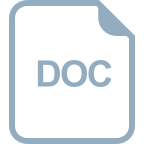


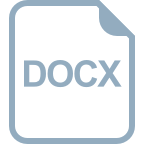
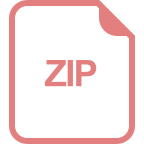






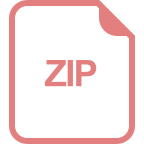
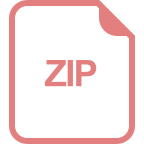
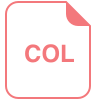


