c语言中{double a[15],k; k=fun(a);},为什么double fun(double a)是错误的fun函数首部
时间: 2024-03-17 18:43:56 浏览: 17
在这段代码中,`double a[15]` 表示定义了一个长度为15的 `double` 类型的数组 `a`,而 `k` 是一个 `double` 类型的变量。`fun(a)` 表示调用函数 `fun`,并将数组 `a` 作为参数传递给函数。
而 `double fun(double a)` 这个函数首部是错误的,因为在这个函数中,参数 `a` 是一个 `double` 类型的变量,而不是一个 `double` 类型的数组。如果要定义一个函数 `fun`,并要求它接受一个长度为15的 `double` 类型的数组作为参数,应该这样定义函数首部:
```
double fun(double a[15])
```
或者
```
double fun(double *a)
```
这两种方式都可以定义一个函数 `fun`,并要求它接受一个长度为15的 `double` 类型的数组作为参数。在函数体中,可以通过 `a[i]` 或者 `*(a+i)` 的方式来访问数组 `a` 中的元素。
相关问题
编写自定义函数fun,计算实数a、b的平方根之和C语言
以下是一个计算实数a、b平方根之和的自定义函数fun的C语言代码:
```c
#include <stdio.h>
#include <math.h>
double fun(double a, double b) {
double sqrt_a = sqrt(a);
double sqrt_b = sqrt(b);
double sum = sqrt_a + sqrt_b;
return sum;
}
int main() {
double a = 2.0, b = 3.0;
double result = fun(a, b);
printf("The square root sum of %lf and %lf is %lf\n", a, b, result);
return 0;
}
```
在上面的代码中,我们定义了一个自定义函数fun,该函数接受两个实数参数a和b,并计算它们的平方根之和。函数中,我们首先使用math.h头文件中的sqrt函数分别计算a和b的平方根,然后将它们相加并返回结果。在main函数中,我们初始化了a和b的值,并调用fun函数来计算它们的平方根之和。最后,我们使用printf函数输出结果。
C语言编程,在主函数中调用fun()计算A(n)=1/{1+A(n-1)},其中A(1)=1
可以这样写一个递归函数来计算A(n):
```c
#include <stdio.h>
double fun(int n) {
if (n == 1) {
return 1;
} else {
return 1.0 / (1.0 + fun(n - 1));
}
}
int main() {
int n = 5; // 假设计算A(5)
double result = fun(n);
printf("A(%d) = %f\n", n, result);
return 0;
}
```
输出结果为:
```
A(5) = 0.618033
```
相关推荐
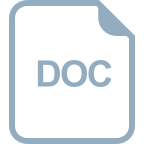
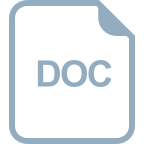
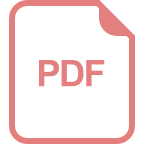












