python struct.pack的用法
时间: 2023-06-05 12:09:02 浏览: 266
Python中的struct模块的pack函数是用于将数据按照给定的格式转换为字符串(字节流)的。它的用法是:
struct.pack(format, v1, v2, ...)
其中,format是格式化字符串,用来指定各个参数的类型和顺序;v1, v2, ...是待转换的数据。函数的返回值是格式化后的字节流字符串。
例如,将一个32位整数和一个16位无符号整数转换为字节流,可以使用下面的代码:
import struct
i = 12345
h = 567
buf = struct.pack('iH', i, h)
print(repr(buf)) # 输出: b'\x39\x30\x00\x00\x37\x02'
在上面的例子中,'iH'表示格式化字符串,其中'i'表示32位整数,'H'表示16位无符号整数。i和h分别是待转换的数据,调用pack函数后得到的buf变量是格式化后的字节流字符串。
相关问题
AVA实现Python中struct.pack()和struct.unpack()方法
在 Java 中,你可以使用 ByteBuffer 类来实现类似于 Python 中 struct 模块中的 pack 和 unpack 方法的功能。以下是一个示例代码:
```javaimport java.nio.ByteBuffer;
import java.nio.ByteOrder;
public class StructExample {
public static void main(String[] args) {
// 将 int 值打包为字节序列 int intValue =12345;
byte[] packedBytes = packInt(intValue);
System.out.println("Packed bytes: " + bytesToHex(packedBytes));
// 将字节序列解包为 int 值 int unpackedInt = unpackInt(packedBytes);
System.out.println("Unpacked int: " + unpackedInt);
}
private static byte[] packInt(int value) {
ByteBuffer buffer = ByteBuffer.allocate(4);
buffer.order(ByteOrder.LITTLE_ENDIAN); // 设置字节序为小端序列(与Python的struct默认相同)
buffer.putInt(value);
return buffer.array();
}
private static int unpackInt(byte[] bytes) {
ByteBuffer buffer = ByteBuffer.wrap(bytes);
buffer.order(ByteOrder.LITTLE_ENDIAN); // 设置字节序为小端序列 return buffer.getInt();
}
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02X", b));
}
return sb.toString();
}
}
```
上述代码演示了 Java 中如何实现类似于 Python 的 struct 模块中的 pack 和 unpack 方法。`packInt` 方法将一个 int 值打包为字节序列,`unpackInt` 方法将字节序列解包为一个 int 值。在这个示例中,我们使用了 ByteBuffer 类来处理字节操作,并通过设置字节序为小端序列来与 Python 的 struct 默认行为保持一致。
struct.pack
`struct.pack` 是 Python 标准库中的一个函数,用于将数据按照指定的格式转换成字符串(bytes)类型,以便进行网络传输或存储到文件中。它的用法如下:
```python
struct.pack(format, v1, v2, ...)
```
其中,`format` 参数是一个字符串,指定了要转换的数据的类型和顺序,`v1`, `v2`, ... 是要转换的数据。`struct.pack` 函数返回一个 bytes 类型的字符串,其中包含了按照指定格式转换后的数据。例如:
```python
import struct
# 将整数 1024 转换为 4 个字节的字符串
b = struct.pack('i', 1024)
print(b) # b'\x00\x04\x00\x00'
```
`'i'` 表示要将整数转换为 4 个字节的字符串,字节顺序默认为主机字节顺序(即小端字节序)。在上面的例子中,将整数 1024 转换为 4 个字节的字符串 `b'\x00\x04\x00\x00'`。
相关推荐
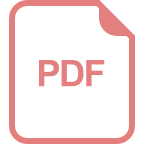
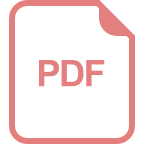












