Ble蓝牙4.2协议传输完整用例
时间: 2023-07-08 17:42:55 浏览: 155
以下是一个简单的Ble蓝牙4.2协议传输完整用例:
1. 初始化设备并扫描周围的Ble设备。
2. 连接到所需的Ble设备。
3. 发现所需的服务和特征。
4. 启用所需的通知特征以接收数据。
5. 发送数据到写入特征。
6. 监听接收到的数据并进行处理。
7. 断开连接。
下面是一个示例代码片段:
```python
# 导入必要的模块和库
from bluepy.btle import Scanner, Peripheral, UUID
# 设置所需的UUID和地址
service_uuid = UUID("0000180f-0000-1000-8000-00805f9b34fb")
char_uuid = UUID("00002a19-0000-1000-8000-00805f9b34fb")
device_address = "00:11:22:33:44:55"
# 扫描设备
scanner = Scanner()
devices = scanner.scan(5.0)
for dev in devices:
if dev.addr == device_address:
# 连接到设备
device = Peripheral(device_address)
# 发现所需的服务和特征
service = device.getServiceByUUID(service_uuid)
characteristic = service.getCharacteristics(char_uuid)[0]
# 启用通知特征以接收数据
characteristic.write(b"\x01\x00")
while True:
# 监听接收到的数据并进行处理
if device.waitForNotifications(1.0):
continue
print("Waiting...")
# 断开连接
device.disconnect()
```
在上面的示例中,我们使用了Python的bluepy库来实现Ble蓝牙4.2协议传输完整用例。我们首先使用Scanner类扫描周围的设备,然后使用Peripheral类连接到所需的设备,并使用getServiceByUUID()和getCharacteristics()方法发现所需的服务和特征。接下来,我们使用write()方法启用通知特征以接收数据,并使用waitForNotifications()方法监听接收到的数据并进行处理。最后,我们使用disconnect()方法断开连接。
相关推荐
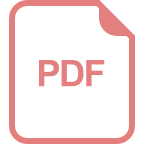














