给我写一个图书管理系统java和mysql
时间: 2024-03-05 20:48:50 浏览: 57
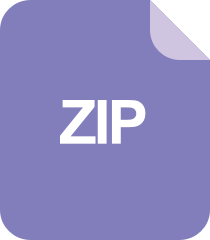
图书管理系统(java+mysql)

好的,以下是一个基于Java和MySQL的图书管理系统的示例代码,包括数据库表结构和数据访问层的实现。
Java代码:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class BookManagementSystem {
private static final String DB_URL = "jdbc:mysql://localhost:3306/bookstore?useSSL=false&serverTimezone=UTC";
private static final String DB_USER = "root";
private static final String DB_PASSWORD = "password";
// 查询所有图书
public static List<Book> getAllBooks() {
List<Book> books = new ArrayList<>();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
stmt = conn.createStatement();
String sql = "SELECT * FROM books";
rs = stmt.executeQuery(sql);
while (rs.next()) {
Book book = new Book();
book.setId(rs.getInt("id"));
book.setTitle(rs.getString("title"));
book.setAuthor(rs.getString("author"));
book.setGenre(rs.getString("genre"));
book.setPrice(rs.getDouble("price"));
books.add(book);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
closeConnection(conn, stmt, rs);
}
return books;
}
// 根据ID查询图书
public static Book getBookById(int id) {
Book book = null;
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "SELECT * FROM books WHERE id = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
rs = pstmt.executeQuery();
if (rs.next()) {
book = new Book();
book.setId(rs.getInt("id"));
book.setTitle(rs.getString("title"));
book.setAuthor(rs.getString("author"));
book.setGenre(rs.getString("genre"));
book.setPrice(rs.getDouble("price"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
closeConnection(conn, pstmt, rs);
}
return book;
}
// 添加图书
public static void addBook(Book book) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "INSERT INTO books(title, author, genre, price) VALUES (?, ?, ?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getGenre());
pstmt.setDouble(4, book.getPrice());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
closeConnection(conn, pstmt, null);
}
}
// 更新图书
public static void updateBook(Book book) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "UPDATE books SET title = ?, author = ?, genre = ?, price = ? WHERE id = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getGenre());
pstmt.setDouble(4, book.getPrice());
pstmt.setInt(5, book.getId());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
closeConnection(conn, pstmt, null);
}
}
// 删除图书
public static void deleteBook(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "DELETE FROM books WHERE id = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
closeConnection(conn, pstmt, null);
}
}
// 关闭数据库连接
private static void closeConnection(Connection conn, Statement stmt, ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
Book类:
```java
public class Book {
private int id;
private String title;
private String author;
private String genre;
private double price;
public Book() {
}
public Book(int id, String title, String author, String genre, double price) {
this.id = id;
this.title = title;
this.author = author;
this.genre = genre;
this.price = price;
}
// 省略getter和setter方法
}
```
MySQL数据库表结构:
```sql
CREATE TABLE IF NOT EXISTS books (
id INT(11) NOT NULL AUTO_INCREMENT,
title VARCHAR(255) NOT NULL,
author VARCHAR(255) NOT NULL,
genre VARCHAR(255) NOT NULL,
price DOUBLE NOT NULL,
PRIMARY KEY (id)
);
```
该系统采用了基于PreparedStatement的数据访问方式,能够有效地防止SQL注入攻击。同时,该系统提供了添加、查询、更新和删除图书的功能,可以根据需要进行扩展。
阅读全文
相关推荐
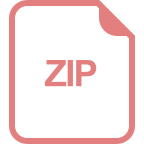
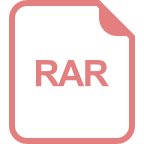
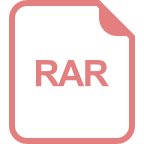
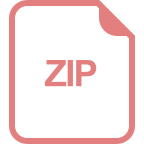
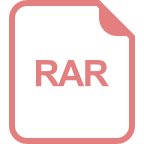
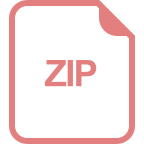
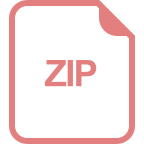
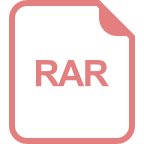
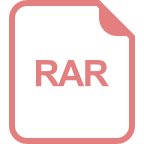
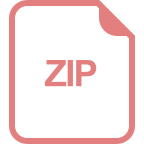
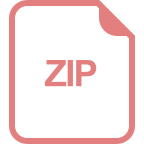
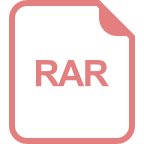
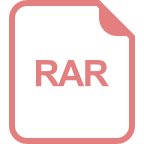
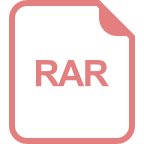