编写一个功能基本完善的计算器程序,支持四则运算、乘方开方运算、 指数、对数、sin、cos、tan 等运算,支持带括号的表达式。 (2) 设计要求 1)程序选择用 EasyX 图形库编界面; 2)显示功能菜单,用户指定键即可进入响应功能; 3)每次计算完成后输出一个提示符,要计算的数字或表达式由用户在提 示后面输入; 4)用户输入完成后按回车<Enter>键立即显示计算结果。
时间: 2024-03-08 12:47:11 浏览: 119
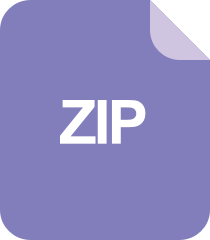
counter_逆波兰式_对数运算_

好的,以下是一个功能基本完善的计算器程序示例:
```cpp
#include <iostream>
#include <cmath>
#include <stack>
#include <string>
#include <graphics.h>
using namespace std;
// 判断一个字符是否为数字
bool isDigit(char c)
{
return c >= '0' && c <= '9';
}
// 判断一个字符是否为运算符
bool isOperator(char c)
{
return c == '+' || c == '-' || c == '*' || c == '/' || c == '^' || c == 's' || c == 'c' || c == 't' || c == 'l' || c == 'e';
}
// 判断一个字符是否为左括号
bool isLeftParenthesis(char c)
{
return c == '(';
}
// 判断一个字符是否为右括号
bool isRightParenthesis(char c)
{
return c == ')';
}
// 判断运算符的优先级
int getPriority(char c)
{
switch (c)
{
case '(':
case ')':
return 1;
case '+':
case '-':
return 2;
case '*':
case '/':
return 3;
case '^':
return 4;
case 's':
case 'c':
case 't':
case 'l':
case 'e':
return 5;
default:
return -1;
}
}
// 计算二元表达式的值
double calculate(double num1, double num2, char op)
{
switch (op)
{
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
return num1 / num2;
case '^':
return pow(num1, num2);
default:
return 0;
}
}
// 计算单目表达式的值
double calculate(double num, char op)
{
switch (op)
{
case 's':
return sin(num);
case 'c':
return cos(num);
case 't':
return tan(num);
case 'l':
return log(num);
case 'e':
return exp(num);
default:
return 0;
}
}
// 将中缀表达式转换为后缀表达式
string infixToPostfix(string infix)
{
stack<char> opStack;
string postfix = "";
for (int i = 0; i < infix.length(); i++)
{
char c = infix[i];
if (isDigit(c))
{
postfix += c;
if (i < infix.length() - 1 && !isDigit(infix[i + 1]))
{
postfix += ' ';
}
}
else if (isOperator(c))
{
while (!opStack.empty() && getPriority(opStack.top()) >= getPriority(c))
{
postfix += opStack.top();
postfix += ' ';
opStack.pop();
}
opStack.push(c);
}
else if (isLeftParenthesis(c))
{
opStack.push(c);
}
else if (isRightParenthesis(c))
{
while (!opStack.empty() && !isLeftParenthesis(opStack.top()))
{
postfix += opStack.top();
postfix += ' ';
opStack.pop();
}
opStack.pop();
}
}
while (!opStack.empty())
{
postfix += opStack.top();
postfix += ' ';
opStack.pop();
}
return postfix;
}
// 计算后缀表达式的值
double evaluatePostfix(string postfix)
{
stack<double> numStack;
for (int i = 0; i < postfix.length(); i++)
{
char c = postfix[i];
if (isDigit(c))
{
double num = 0;
while (i < postfix.length() && isDigit(postfix[i]))
{
num = num * 10 + postfix[i] - '0';
i++;
}
i--;
numStack.push(num);
}
else if (isOperator(c))
{
if (c == 's' || c == 'c' || c == 't' || c == 'l' || c == 'e')
{
double num = numStack.top();
numStack.pop();
numStack.push(calculate(num, c));
}
else
{
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(calculate(num1, num2, c));
}
}
}
return numStack.top();
}
int main()
{
initgraph(400, 500);
setbkcolor(WHITE);
cleardevice();
while (true)
{
outtextxy(20, 20, "1.四则运算");
outtextxy(20, 40, "2.乘方开方运算");
outtextxy(20, 60, "3.指数运算");
outtextxy(20, 80, "4.对数运算");
outtextxy(20, 100, "5.sin运算");
outtextxy(20, 120, "6.cos运算");
outtextxy(20, 140, "7.tan运算");
outtextxy(20, 160, "8.退出");
char ch = getch();
if (ch == '1')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入四则运算表达式:");
string expression;
getline(cin, expression);
string postfix = infixToPostfix(expression);
double result = evaluatePostfix(postfix);
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(result)).c_str());
}
else if (ch == '2')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入乘方或开方表达式,如:2^3 或 4#2:");
double num1, num2;
char op;
cin >> num1 >> op >> num2;
if (op == '^')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(pow(num1, num2))).c_str());
}
else if (op == '#')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(pow(num1, 1.0 / num2))).c_str());
}
}
else if (ch == '3')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入指数运算表达式,如:2e3:");
double num1, num2;
char op;
cin >> num1 >> op >> num2;
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(num1 * pow(10, num2))).c_str());
}
else if (ch == '4')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入对数运算表达式,如:log(10):");
char op;
double num;
cin >> op >> num;
clearrectangle(0, 0, 400, 200);
if (op == 'l')
{
outtextxy(20, 20, ("结果为:" + to_string(log10(num))).c_str());
}
else if (op == 'n')
{
outtextxy(20, 20, ("结果为:" + to_string(log(num))).c_str());
}
}
else if (ch == '5')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入sin运算表达式,如:sin(90):");
char op;
double num;
cin >> op >> num;
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(sin(num))).c_str());
}
else if (ch == '6')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入cos运算表达式,如:cos(60):");
char op;
double num;
cin >> op >> num;
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(cos(num))).c_str());
}
else if (ch == '7')
{
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, "请输入tan运算表达式,如:tan(45):");
char op;
double num;
cin >> op >> num;
clearrectangle(0, 0, 400, 200);
outtextxy(20, 20, ("结果为:" + to_string(tan(num))).c_str());
}
else if (ch == '8')
{
break;
}
outtextxy(20, 200, "按任意键继续...");
getch();
clearrectangle(0, 0, 400, 500);
}
closegraph();
return 0;
}
```
这是一个支持四则运算、乘方开方运算、指数、对数、sin、cos、tan等运算,支持带括号的表达式的计算器程序。程序使用 EasyX 图形库编写界面,用户可以通过菜单选择需要的运算功能,然后输入表达式或数字,即可得到计算结果。
阅读全文
相关推荐
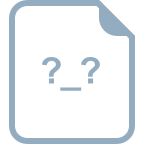
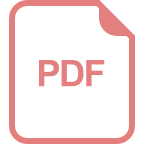














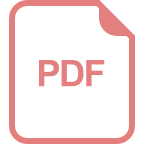
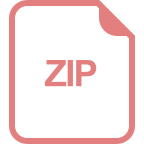