java自定义一个学生类,包含两个属性姓名,成绩。将几个学生对象存入到treeset集合中去
时间: 2024-02-22 08:57:28 浏览: 24
好的,我来给你写一个Java自定义学生类,并将多个学生对象存入TreeSet集合中。
```java
import java.util.*;
public class Student implements Comparable<Student> {
private String name;
private double score;
public Student(String name, double score) {
this.name = name;
this.score = score;
}
public String getName() {
return name;
}
public double getScore() {
return score;
}
// 实现Comparable接口中的compareTo方法,用于TreeSet排序
@Override
public int compareTo(Student o) {
if (this.score > o.score) {
return -1;
} else if (this.score < o.score) {
return 1;
} else {
return this.name.compareTo(o.name);
}
}
public static void main(String[] args) {
Student s1 = new Student("Tom", 85.5);
Student s2 = new Student("Jack", 92.0);
Student s3 = new Student("Lucy", 78.5);
// 将学生对象存入TreeSet集合中
TreeSet<Student> set = new TreeSet<>();
set.add(s1);
set.add(s2);
set.add(s3);
// 遍历TreeSet集合中的学生对象
for (Student s : set) {
System.out.println("姓名:" + s.getName() + ",成绩:" + s.getScore());
}
}
}
```
上述代码中,我们通过实现Comparable接口中的compareTo方法,将学生对象按照成绩从高到低排序,如果成绩相同,则按照姓名首字母的字典序进行排序。接着将多个学生对象存入TreeSet集合中,最后遍历集合中的学生对象,输出学生姓名和成绩。
相关推荐
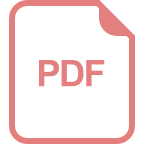
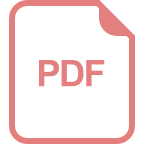
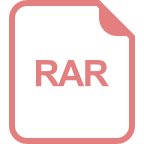














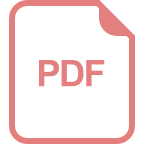