二叉树的非递归遍历c++
时间: 2023-08-29 07:12:21 浏览: 45
二叉树的非递归遍历可以使用栈来实现。以下是 C++ 实现的代码:
```c++
#include <iostream>
#include <stack>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
void preOrderTraversal(TreeNode* root) {
if (root == NULL) return;
stack<TreeNode*> s;
s.push(root);
while (!s.empty()) {
TreeNode* cur = s.top();
s.pop();
cout << cur->val << " ";
if (cur->right) s.push(cur->right);
if (cur->left) s.push(cur->left);
}
}
void inOrderTraversal(TreeNode* root) {
if (root == NULL) return;
stack<TreeNode*> s;
TreeNode* cur = root;
while (cur != NULL || !s.empty()) {
while (cur != NULL) {
s.push(cur);
cur = cur->left;
}
cur = s.top();
s.pop();
cout << cur->val << " ";
cur = cur->right;
}
}
void postOrderTraversal(TreeNode* root) {
if (root == NULL) return;
stack<TreeNode*> s;
TreeNode* cur = root;
TreeNode* last = NULL;
while (cur != NULL || !s.empty()) {
while (cur != NULL) {
s.push(cur);
cur = cur->left;
}
cur = s.top();
if (cur->right == NULL || cur->right == last) {
s.pop();
cout << cur->val << " ";
last = cur;
cur = NULL;
} else {
cur = cur->right;
}
}
}
int main() {
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
root->right->left = new TreeNode(6);
root->right->right = new TreeNode(7);
cout << "Preorder Traversal: ";
preOrderTraversal(root);
cout << endl;
cout << "Inorder Traversal: ";
inOrderTraversal(root);
cout << endl;
cout << "Postorder Traversal: ";
postOrderTraversal(root);
cout << endl;
return 0;
}
```
这里实现了三种遍历方式:前序遍历、中序遍历和后序遍历。其中,前序遍历和中序遍历比较简单,后序遍历需要使用一个 `last` 指针来记录上一个被访问的结点,以判断当前结点是否可以被访问。
相关推荐
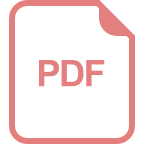














