const person = { firstName:" John", lastName:" Doe", age: 25 }; for (let x in person) { txt += person[x]; }
时间: 2024-03-29 07:35:17 浏览: 93
这是一段 JavaScript 代码,其中定义了一个名为 `person` 的常量,它是一个对象,具有三个属性:`firstName`、`lastName` 和 `age`。接下来,使用 for-in 循环遍历了 `person` 对象的属性,并将每个属性的值累加到一个名为 `txt` 的变量中。最终,`txt` 变量中存储了所有属性的值组成的字符串。需要注意的是,在 for-in 循环中,变量 x 代表的是属性名,而不是属性值。如果要获取属性值,需要使用 `person[x]` 的形式。
相关问题
const person = {firstName: "John", lastName: "Doe", age: 30}; for (const key in person) { console.log(`${key}: ${person[key]}`); }
这段代码会输出对象 `person` 中的每个属性及其对应的值。具体来说,循环会遍历对象 `person` 的所有属性,将每个属性的名称赋值给变量 `key`,然后通过 `${key}: ${person[key]}` 的方式将属性名和属性值拼接成一个字符串,最后通过 `console.log()` 函数输出这个字符串。
假设你运行这段代码,它会输出:
```
firstName: John
lastName: Doe
age: 30
```
这是因为对象 `person` 有三个属性,分别是 `firstName`、`lastName` 和 `age`,循环会分别遍历这三个属性,并输出每个属性的名称和值。
修改下列代码使其能在 Person 原型上挂载 print 方法,并在 print 方法内输出 first, last, age, eyecolor 四项参数的值,格式任意const Person = (first, last, age, eyecolor) => { this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eyecolor; }
要在 Person 原型上挂载 print 方法,可以使用如下代码:
```javascript
function Person(first, last, age, eyecolor) {
this.firstName = first;
this.lastName = last;
this.age = age;
this.eyeColor = eyecolor;
}
Person.prototype.print = function() {
console.log(this.firstName, this.lastName, this.age, this.eyeColor);
}
const person = new Person('John', 'Doe', 30, 'blue');
person.print(); // 输出 John Doe 30 blue
```
在上面的代码中,我们使用了函数声明的方式定义了 Person 类。然后,我们使用 Person.prototype 对象来添加 print 方法,该方法可以访问实例的属性。最后,我们创建了一个 Person 实例,并调用 print 方法来输出属性的值。
阅读全文
相关推荐
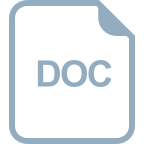
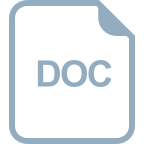
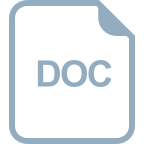
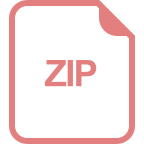
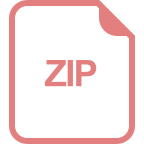
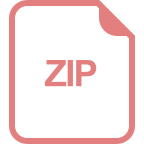
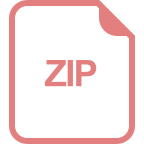
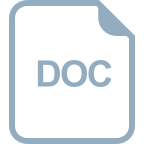
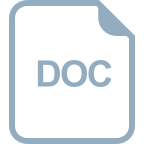
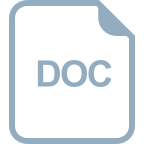
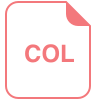
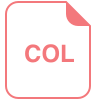
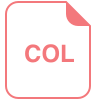
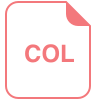
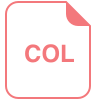
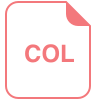
