C++编写一个程序实现查找重复数字的功能。数组a中存放了10个随机整数(1-10),要求用指针p和q分别指向需要比较的单元,并使每个重复的数字只出现一次
时间: 2024-12-16 14:29:48 浏览: 7
在C++中,我们可以创建一个简单的程序来实现这个功能。下面是一个基本的示例,使用两个指针`p`和`q`遍历数组,检查是否有重复的数字。这里假设我们有一个名为`findDuplicates`的函数:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义一个辅助函数用于交换元素
void swap(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
// 查找并移除重复的数字
bool findAndRemoveDuplicates(vector<int>& arr) {
vector<int>::iterator it1 = arr.begin(), it2 = arr.begin();
while (it1 != arr.end()) {
if (*it1 == *it2) {
// 如果找到重复项,将其从数组中移除并将它和下一个元素交换
swap(*it1, *(++it2));
--it2; // 减小it2,因为它已经移动过了
} else {
++it1; // 没有重复,继续遍历
}
}
return !arr.empty(); // 如果数组非空,说明存在重复
}
int main() {
vector<int> a(10);
// 假设这里通过random_shuffle填充随机整数(1-10)
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> dis(1, 10);
for (int i = 0; i < a.size(); ++i) {
a[i] = dis(gen);
}
// 打印原始数组
cout << "Original array:\n";
for (int num : a) {
cout << num << " ";
}
cout << endl;
if (findAndRemoveDuplicates(a)) {
cout << "Array after removing duplicates:\n";
for (int num : a) {
cout << num << " ";
}
} else {
cout << "No duplicate numbers found.\n";
}
return 0;
}
```
在这个例子中,`findAndRemoveDuplicates`函数会遍历数组,如果发现相同的数字,就利用`swap`函数将其替换为后面的元素。这个过程会一直持续到数组中不再有重复数字为止。
阅读全文
相关推荐
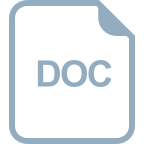
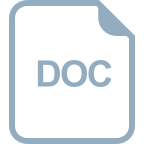



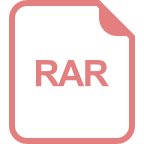
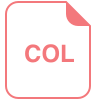
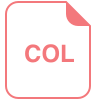
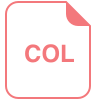
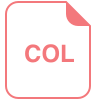
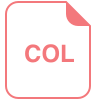
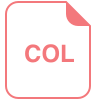
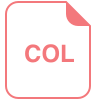
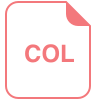
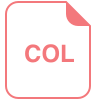
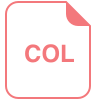
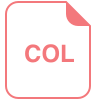
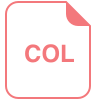
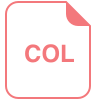