c语言请按尾插法创建单链表,并在指定位置插入元素,输出插入完成后的链表输入包括三行第一行输入单链表的长度第二行输入单链表的元素第三行输入插入的位置和插入的值输出插入完成后的单链表,若插入失败,输出-1。
时间: 2024-09-16 08:03:51 浏览: 41
在C语言中,你可以使用结构体表示链表节点,并通过按尾插法在给定位置插入元素。以下是步骤:
首先,定义链表节点结构:
```c
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
然后,编写函数实现链表操作:
```c
// 创建新节点并插入到链表尾部
Node* insertAtTail(Node** head, int value) {
if (*head == NULL) { // 如果链表为空,新建头节点
*head = (Node*)malloc(sizeof(Node));
(*head)->data = value;
(*head)->next = NULL;
return *head;
}
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
// 在链表尾部插入新的节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
current->next = newNode;
return *head;
}
// 插入元素到指定位置(从0开始计数)
Node* insertAtPosition(Node** head, int position, int value) {
if (position <= 0 || position > lengthOfList(*head)) {
printf("-1\n"); // 插入失败,输出-1
return NULL;
}
Node* newHead = *head;
Node* prev = NULL;
for (int i = 0; i < position - 1 && newHead != NULL; i++) {
prev = newHead;
newHead = newHead->next;
}
if (newHead == NULL) {
return insertAtTail(head, value); // 如果已到达尾部,像尾插法一样插入
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = newHead;
prev->next = newNode;
return newHead;
}
```
这里的`lengthOfList()`函数需要你自己实现,它用于获取链表的实际长度。
最后,在主程序中调用上述函数处理用户输入:
```c
int main() {
int listLength, element, position;
scanf("%d", &listLength);
Node* head = NULL;
// 读取链表元素
for (int i = 0; i < listLength; i++) {
scanf("%d", &element);
head = insertAtTail(&head, element);
}
// 用户指定插入位置和值
scanf("%d %d", &position, &value);
// 插入元素到指定位置
Node* newHead = insertAtPosition(&head, position, value);
if (newHead == NULL) {
return 0;
}
// 输出插入后的链表
printLinkedList(newHead);
return 0;
}
```
注意,这里假设`printLinkedList()`是一个自定义函数,用于打印链表内容。你需要编写这个辅助函数来显示链表。
阅读全文
相关推荐
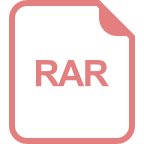
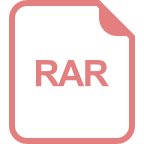
















