#include<iostream> #include<vector> using namespace std; vector<int> x,x1;//定义动态数组 vector<vector<int> > p; int powermax = -1; int n; //判断角色k的位置是否可行 bool position(int k){ for(int i = 1;i < k;i ++){ if(x[k] == x[i]){ return false; } } return true; } //回溯法求最优解 void backTrack(int t){ if(t > n){//求总攻击力并更新最大值 int power = 0; for(int i = 1;i <= n;i ++){ power = power + p[i][x[i]]; } if(power > powermax){ powermax = power; x1 = x;//记录当前最优解的排列 } }else{//遍历可行排列 for(int i = 1;i <= n;i ++){ x[t] = i; if(position(t)){ backTrack(t+1); } } } } int main(){ cout<<"请输入兵种个数:\n"; cin >> n; for(int i = 0;i <= n;i ++){ x.push_back(0); x1.push_back(0); } for(int i = 0;i <= n;i ++){ p.push_back(x); } for(int i = 1;i <= n;i ++){ for(int j = 1;j <= n;j ++){ printf("请输入第%d个兵种在第%d个位置的攻击力:\n",i,j); int p1; cin >> p1; p[i][j] = p1; } } backTrack(1); cout << "最优解为:" << endl; for(int i = 1;i <= n;i ++){ cout << x1[i] << " "; } cout << "最大攻击力为:" << powermax << endl; return 0; }给出该程序算法实际思想流程
时间: 2024-04-27 08:25:20 浏览: 123
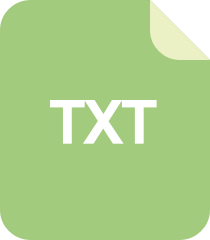
使用include <iostream>时一定要加using namespace std
这是一个使用回溯法求解兵种排列的程序。具体来说,它的算法思想流程如下:
1、首先输入兵种个数 n。
2、然后使用动态数组 vector 存储兵种的攻击力和排列。
3、接着使用嵌套循环输入每个兵种在每个位置的攻击力。
4、定义一个 position 函数,用于判断某个兵种在某个位置是否可行。如果该位置已经有其他兵种,则返回 false,否则返回 true。
5、定义一个 backTrack 函数,使用回溯法求出最优解。
6、在 backTrack 函数中,如果 t > n,表示已经遍历完了所有兵种的排列,此时求出总攻击力并更新最大值。同时记录当前最优解的排列。
7、如果 t <= n,表示还未遍历完所有兵种的排列,此时遍历可行排列,如果该排列可行,则继续递归调用 backTrack 函数。
8、最后输出最优解的排列和最大攻击力。
总的来说,该程序的算法思想流程是使用回溯法枚举所有可能的兵种排列,然后找出其中最优解。
阅读全文
相关推荐
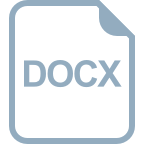

















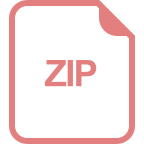