图卷积gcn的应用实例
时间: 2023-11-02 17:02:45 浏览: 47
图卷积网络(Graph Convolutional Networks,GCN)是一种用于处理图结构数据的深度学习模型。GCN的应用实例如下:
1. 社交网络分析:社交网络是一个典型的图结构数据,GCN可以用于社交网络的社区发现、节点分类和链接预测等任务。例如可以利用GCN来发现社交网络中的子群体,推荐用户或商品,预测用户的兴趣等。
2. 蛋白质互作网络分析:蛋白质之间的相互作用关系可以表示为图结构数据。GCN可以用于蛋白质之间的互作预测,研究蛋白质相互作用网络的拓扑结构和功能。
3. 推荐系统:GCN可以用于推荐系统中的用户-物品交互行为建模。传统的协同过滤方法无法直接考虑用户和物品之间的图结构关系,而GCN可以充分利用图结构信息提高推荐准确性。
4. 遥感图像分析:GCN可以用于处理遥感图像数据中的地理空间关系。通过利用图卷积神经网络,可以实现遥感图像的目标检测、地物分类和变化检测等任务。
总之,GCN的应用范围广泛,可以应用于各种需要处理图结构数据的任务,如社交网络分析、蛋白质互作网络分析、推荐系统和遥感图像分析等。通过学习图结构的相互关系,GCN能够在这些任务中提供更准确和有效的结果。
相关问题
图卷积网络(GCN)代码
以下是使用PyTorch实现的简单图卷积网络(GCN)代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class GCNLayer(nn.Module):
def __init__(self, in_channels, out_channels):
super(GCNLayer, self).__init__()
self.linear = nn.Linear(in_channels, out_channels)
def forward(self, x, adj):
# x: input features (batch_size, num_nodes, in_channels)
# adj: adjacency matrix (batch_size, num_nodes, num_nodes)
x = self.linear(x)
adj = adj + torch.eye(adj.size(1)).unsqueeze(0).to(adj.device) # add self-loop
degree = torch.sum(adj, dim=2, keepdim=True)
adj = adj / degree # normalize adjacency matrix
x = torch.bmm(adj, x)
return F.relu(x)
class GCN(nn.Module):
def __init__(self, in_channels, hidden_channels, out_channels):
super(GCN, self).__init__()
self.layer1 = GCNLayer(in_channels, hidden_channels)
self.layer2 = GCNLayer(hidden_channels, out_channels)
def forward(self, x, adj):
x = self.layer1(x, adj)
x = self.layer2(x, adj)
return x
```
以上代码实现了一个包含两个GCN层的GCN模型,可以通过调用`GCN(in_channels, hidden_channels, out_channels)`来实例化该模型。其中,`in_channels`是输入特征的维度,`hidden_channels`是隐藏层的维度,`out_channels`是输出特征的维度。在模型的`forward`函数中,首先通过`GCNLayer`层对输入特征进行线性变换和非线性激活,然后根据邻接矩阵进行卷积操作,最终输出特征。
图卷积网络进行骨骼识别代码_PyG训练图卷积网络GCN的代码参考
以下是一个使用Pytorch Geometric (PyG)库训练图卷积网络(GCN)进行骨骼识别的代码示例:
```python
import torch
import torch.nn.functional as F
from torch_geometric.datasets import Human36M
from torch_geometric.nn import GCNConv
# 加载数据集
train_dataset = Human36M(root='/path/to/dataset', train=True)
test_dataset = Human36M(root='/path/to/dataset', train=False)
# 定义图卷积网络模型
class GCN(torch.nn.Module):
def __init__(self):
super(GCN, self).__init__()
self.conv1 = GCNConv(54, 128) # 第一层GCN卷积
self.conv2 = GCNConv(128, 128) # 第二层GCN卷积
self.fc1 = torch.nn.Linear(128, 64) # 全连接层
self.fc2 = torch.nn.Linear(64, 32) # 全连接层
self.fc3 = torch.nn.Linear(32, 17) # 全连接层
def forward(self, x, edge_index):
# x: 特征向量
# edge_index: 图的邻接矩阵
x = F.relu(self.conv1(x, edge_index)) # GCN卷积层1
x = F.relu(self.conv2(x, edge_index)) # GCN卷积层2
x = F.relu(self.fc1(x)) # 全连接层1
x = F.relu(self.fc2(x)) # 全连接层2
x = self.fc3(x) # 全连接层3, 输出17维向量
return x
# 实例化模型
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = GCN().to(device)
# 定义损失函数和优化器
criterion = torch.nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# 训练模型
model.train()
for epoch in range(50):
train_loss = 0.0
for batch in train_dataset:
x, edge_index, y = batch.x.to(device), batch.edge_index.to(device), batch.y.to(device)
optimizer.zero_grad()
out = model(x, edge_index)
loss = criterion(out, y)
loss.backward()
optimizer.step()
train_loss += loss.item() * batch.num_graphs
train_loss /= len(train_dataset)
print('Epoch: {:03d}, Train Loss: {:.7f}'.format(epoch, train_loss))
# 测试模型
model.eval()
test_loss = 0.0
for batch in test_dataset:
x, edge_index, y = batch.x.to(device), batch.edge_index.to(device), batch.y.to(device)
out = model(x, edge_index)
loss = criterion(out, y)
test_loss += loss.item() * batch.num_graphs
test_loss /= len(test_dataset)
print('Test Loss: {:.7f}'.format(test_loss))
```
在这个示例中,我们使用了Human3.6M数据集进行骨骼识别。该数据集包含了大量的人体骨骼姿态数据,每个姿态由17个关键点组成。我们使用GCN对每个关键点进行分类,输出17维向量,每一维代表一个关键点的分类得分。我们使用均方误差(MSE)作为损失函数,使用Adam优化器进行优化。在训练过程中,我们使用了50个epoch进行训练,每个epoch中遍历整个训练集。在测试过程中,我们仅仅计算了测试集上的损失,没有进行预测。
相关推荐
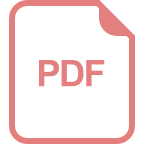












