mybatis雪花算法工具类
时间: 2023-09-13 12:08:54 浏览: 74
Sure! Here's an example implementation of a MyBatis Snowflake algorithm utility class in Java:
```java
import java.util.Calendar;
public class SnowflakeUtils {
private final static long START_STAMP = 1480166465631L;
private final static long SEQUENCE_BIT = 12;
private final static long MACHINE_BIT = 10;
private final static long MAX_SEQUENCE = -1L ^ (-1L << SEQUENCE_BIT);
private final static long MACHINE_ID = getMachineId();
private static long lastTimestamp = -1L;
private static long sequence = 0L;
private static synchronized long nextId() {
long timestamp = timeGen();
if (timestamp < lastTimestamp) {
throw new RuntimeException("Clock moved backwards. Refusing to generate id.");
}
if (timestamp == lastTimestamp) {
sequence = (sequence + 1) & MAX_SEQUENCE;
if (sequence == 0) {
timestamp = tilNextMillis(lastTimestamp);
}
} else {
sequence = 0L;
}
lastTimestamp = timestamp;
return ((timestamp - START_STAMP) << (SEQUENCE_BIT + MACHINE_BIT)) | (MACHINE_ID << SEQUENCE_BIT) | sequence;
}
private static long tilNextMillis(long lastTimestamp) {
long timestamp = timeGen();
while (timestamp <= lastTimestamp) {
timestamp = timeGen();
}
return timestamp;
}
private static long timeGen() {
return Calendar.getInstance().getTimeInMillis();
}
private static long getMachineId() {
// Replace this with logic to get a unique machine id
return 1L;
}
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
System.out.println("Generated ID: " + nextId());
}
}
}
```
This utility class implements the Snowflake algorithm, which is a widely-used algorithm for generating unique IDs in a distributed system. The `nextId()` method generates the IDs based on the configured machine id and the current timestamp.
Please note that the `getMachineId()` method needs to be implemented according to your specific environment to obtain a unique machine id. This can be done based on the network configuration of your machines, or you can use other methods such as reading a hardware identifier.
You can run the `main` method to test the implementation and see generated IDs.
相关推荐
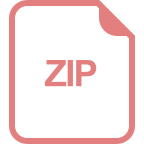
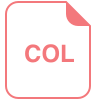
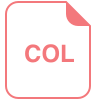
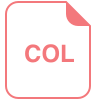
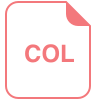










