用C加加语言写出1、利用头插法或尾插法建立单链表。
时间: 2024-09-26 20:14:04 浏览: 9
在C语言中,我们可以使用结构体来表示链表节点,并通过指针来实现头插法或尾插法构建链表。以下是两个基本的示例:
### 首先,定义链表节点结构体:
```c
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
#### 1. 头插法 (Prepend) - 插入到链表头部:
```c
void prepend(Node** head_ref, int new_data) {
Node* new_node = (Node*)malloc(sizeof(Node)); // 分配内存
if (new_node == NULL) { // 内存分配失败处理
printf("Memory allocation failed.\n");
return;
}
new_node->data = new_data; // 设置新节点的数据
new_node->next = *head_ref; // 将新的头指向现有头
*head_ref = new_node; // 更新链表头指针
}
```
#### 2. 尾插法 (Append) - 插入到链表尾部:
```c
void append(Node** head_ref, int new_data) {
Node* new_node = (Node*)malloc(sizeof(Node));
if (new_node == NULL) {
printf("Memory allocation failed.\n");
return;
}
new_node->data = new_data;
new_node->next = NULL;
if (*head_ref == NULL) { // 如果链表为空
*head_ref = new_node;
} else {
Node* temp = *head_ref;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = new_node; // 新节点链接到最后一个节点之后
}
}
```
相关推荐
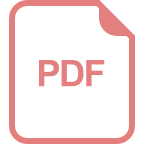
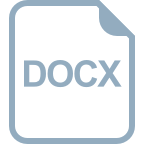
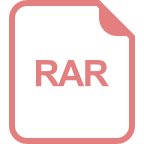














