resultMap什么时候会用到这个
时间: 2024-01-27 15:38:32 浏览: 20
resultMap是MyBatis中一种用来映射查询结果集的方式,通常用于处理复杂查询结果,或者处理查询结果与对象属性不一致的情况。使用resultMap可以定义查询结果集中每个列与Java对象属性的对应关系,以便MyBatis可以自动将查询结果映射到Java对象中。通常在查询结果集比较复杂,需要进行多表关联查询或者需要处理一些特殊情况时,会使用resultMap。
相关问题
mybatis resultmap association 多个参数
### 回答1:
在 MyBatis 中,可以通过 `association` 标签将两个表或实体类关联起来。当查询结果包含两个或多个表的数据时,可以使用 `association` 标签将这些数据关联起来。
如果你想在 `association` 中传递多个参数,可以使用 `parameterType` 属性指定一个自定义的参数类型,然后在 SQL 语句中使用 `${property.field1}, ${property.field2}, ...` 的方式引用这些参数。
举个例子,假设我们有两个实体类 `Order` 和 `User`,它们之间存在一对多的关系,一个用户可以有多个订单。我们可以通过以下方式配置 `resultMap`:
```xml
<resultMap id="orderResultMap" type="Order">
<id property="id" column="order_id" />
<result property="orderNo" column="order_no" />
<result property="userId" column="user_id" />
<association property="user" javaType="User" resultMap="userResultMap">
<id property="id" column="user_id" />
<result property="username" column="username" />
<result property="email" column="email" />
</association>
</resultMap>
<resultMap id="userResultMap" type="User">
<id property="id" column="user_id" />
<result property="username" column="username" />
<result property="email" column="email" />
</resultMap>
```
在 SQL 语句中,我们可以使用 `${param.field1}, ${param.field2}, ...` 的方式引用这些参数,如下所示:
```xml
<select id="getOrderByUserId" resultMap="orderResultMap">
SELECT o.order_id, o.order_no, o.user_id, u.username, u.email
FROM orders o
LEFT JOIN users u ON o.user_id = u.user_id
WHERE o.user_id = #{userId}
</select>
```
在 Java 代码中,我们可以通过 `Map` 或自定义类型的方式传递多个参数,例如:
```java
public interface OrderMapper {
List<Order> getOrderByUserId(Map<String, Object> params);
List<Order> getOrderByUserId(OrderQuery query);
}
public class OrderQuery {
private Long userId;
private String field1;
private String field2;
// getter/setter 略
}
```
使用 `Map` 传递参数的话,可以将多个参数打包成一个 `Map`,然后将该 `Map` 传入 SQL 语句。使用自定义类型的方式传递参数的话,需要在 `parameterType` 属性中指定该类型,例如:
```xml
<select id="getOrderByUserId" resultMap="orderResultMap" parameterType="com.example.OrderQuery">
SELECT o.order_id, o.order_no, o.user_id, u.username, u.email
FROM orders o
LEFT JOIN users u ON o.user_id = u.user_id
WHERE o.user_id = #{userId}
AND o.field1 = #{field1}
AND o.field2 = #{field2}
</select>
```
### 回答2:
Mybatis中的ResultMap Association用于处理多个参数的情况。当我们在查询过程中需要用到多个参数时,可以使用ResultMap Association来将多个参数关联起来,以方便我们进行查询操作。
首先,在Mapper.xml文件中定义ResultMap,使用<resultMap>标签来定义关联关系。在<resultMap>标签的子标签<association>中,我们可以设置关联的多个参数及其对应的属性。
例如,假设我们有两个参数,一个是User对象的id,另一个是Order对象的orderId。那么我们可以使用如下的ResultMap来定义关联关系:
<resultMap id="userOrderResultMap" type="User">
<id property="id" column="user_id"/>
<association property="order" javaType="Order">
<id property="orderId" column="order_id"/>
</association>
</resultMap>
在这个ResultMap中,我们将User对象的id属性与"user_id"字段进行了映射,并将Order对象的orderId属性与"order_id"字段进行了映射。通过关联关系,我们可以在查询过程中同时获取到User对象和Order对象的相关属性。
接下来,我们可以在Mapper接口的方法中使用这个ResultMap来进行查询操作。在查询语句中,我们可以使用关联的多个参数,如#{id}和#{orderId}。
例如,假设我们需要根据用户id和订单id查询订单详情,那么可以使用如下的SQL语句:
<select id="getUserOrderDetail" resultMap="userOrderResultMap">
SELECT * FROM user u
JOIN order o ON u.id = o.user_id
WHERE u.id = #{id} AND o.order_id = #{orderId}
</select>
这样,当我们调用getUserOrderDetail方法时,将传入id和orderId两个参数,Mybatis会根据这些参数进行查询,并将查询结果封装成User对象和关联的Order对象返回。
总之,使用Mybatis的ResultMap Association可以方便地处理多个参数的情况,通过定义关联关系,我们可以在查询过程中同时获取到多个参数的相关属性。
### 回答3:
mybatis的resultmap association可以用于映射多个参数。
在使用resultmap association时,需要创建一个主resultmap和一个关联的resultmap。主resultmap用于映射主对象的属性,而关联的resultmap用于映射关联对象的属性。
首先,我们需要在主resultmap中定义一个association元素,用于关联对象的映射。在association元素中,我们可以指定一个property属性,用于指定主对象中关联对象的属性名称;同时,我们还需要指定一个javaType属性,用于指定关联对象的类型;此外,我们还可以在association元素中定义id元素和result元素,用于映射关联对象的属性。
接下来,在关联的resultmap中,我们可以定义多个id元素和result元素,用于映射关联对象的属性。在id元素和result元素中,我们需要指定一个column属性,用于指定数据库中的列名;同时,我们还需要指定一个property属性,用于指定关联对象中属性的名称。
最后,在使用resultmap association时,我们需要在主resultmap中调用关联的resultmap。可以通过在主resultmap的id元素和result元素中使用association元素的select属性来关联关联的resultmap。在select属性中,我们可以指定一个SQL语句或者一个已经定义的SQL片段的名称,用于查询关联对象的数据。
总而言之,通过使用resultmap association,我们可以将多个参数关联起来,实现复杂的数据映射。
MyBatis中resultMap详解
MyBatis是一种基于XML文件配置的持久化框架,其中resultMap是其中的一个非常重要的组件之一。简单来说,就是将查询结果映射成一个Java对象,以便于程序员的使用和维护。下面就来详细了解一下MyBatis中的resultMap。
1. resultMap的定义
在MyBatis的Mapper XML文件中,可以通过定义resultMap来映射查询结果。具体的定义如下:
```
<resultMap id="resultMapName" type="resultType">
<!-- 这里定义映射关系 -->
</resultMap>
```
其中,id为resultMap的名字,type为结果类型,也就是结果对象所在的类的全限定名。这里需要注意,resultType和type只能设置一个。
2. resultMap中的映射关系
在resultMap中,映射关系定义使用的是result标签,具体定义如下:
```
<result property="propertyName" column="columnName" javaType="javaType" jdbcType="jdbcType" typeHandler="typeHandler" />
```
其中,property为结果对象中的属性名,column为查询语句中的列名,javaType为该属性的Java类型,jdbcType为该属性在数据库中的类型,typeHandler为类型转换器。需要注意的是,column、javaType、jdbcType和typeHandler是可选的,如果没有指定,则MyBatis会自动根据属性名和列名进行简单的映射。
3. 关联关系映射
在实际开发中,经常会涉及到多张表之间的关联查询,此时需要用到MyBatis中的关联映射。具体定义如下:
```
<association property="propertyName" resultMap="resultMapName" />
```
其中,propertyName为当前结果对象中用来保存关联对象的属性名,resultMapName为该关联对象所用的resultMap的名字。
4. 集合关联映射
除了单个对象之外,有时候还需要将查询结果映射成一个包含多个对象的集合。此时需要用到MyBatis中的集合关联映射。具体定义如下:
```
<collection property="propertyName" resultMap="resultMapName" />
```
其中,propertyName为当前结果对象中用来保存集合的属性名,resultMapName为该集合中元素所用的resultMap的名字。
5. resultMap的使用
在映射结果时,需要在查询语句中使用resultMap,具体定义如下:
```
<select id="selectUser" parameterType="int" resultMap="resultMapName">
select id, username, password
from user
where id = #{id}
</select>
```
其中,resultMapName为映射结果所用的resultMap的名字。
综上所述,MyBatis中的resultMap是一个非常重要的组件,能够实现查询结果的映射和关联关系的处理,大大提高了程序员的开发效率。
相关推荐
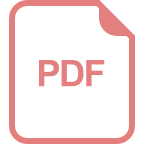
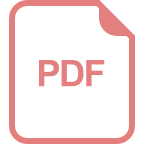
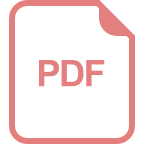











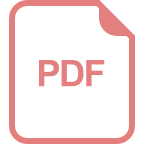