public class _01_ { public static void main(String[] args) { int[] nums = {3,2,4}; int target = 6; } public int[] twoSum(int[] nums, int target) { for (int i = 0; i < nums.length; i++) { for (int j = 0; j < nums.length; j++) { if (nums[i] + nums[j] == target) { return new int[]{i, j}; } } } return new int[]{}; } }这段代码main里面为什么调不到twosum方法
时间: 2024-02-14 17:11:50 浏览: 79
这段代码中的`twoSum`方法是一个非静态方法,必须通过创建一个对象的方式才能调用,而`main`方法是静态方法,不能直接调用非静态方法。因此,在`main`方法中调用`twoSum`方法时需要先创建一个对象,然后通过对象来调用。例如:
```
public class _01_ {
public static void main(String[] args) {
int[] nums = {3,2,4};
int target = 6;
_01_ solution = new _01_(); // 创建对象
int[] result = solution.twoSum(nums, target); // 通过对象调用方法
System.out.println(Arrays.toString(result));
}
public int[] twoSum(int[] nums, int target) {
// 实现方法
}
}
```
相关问题
import java.util.*; public class Main4 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); int[] nums = new int[n]; for (int i = 0; i < n; i++) { nums[i] = sc.nextInt(); } for (int i = 1; i < n; i++) { int temp = nums[i]; int j = i - 1; while (j >= 0 && nums[j] > temp) { nums[j + 1] = nums[j]; j--; } nums[j + 1] = temp; System.out.print(j + 1 + " "); } } }
这段 Java 代码是实现了插入排序,并且在每次插入操作完成后输出当前元素的插入位置。具体来说,代码首先通过 Scanner 类获取输入,然后使用插入排序对输入的数组进行排序。排序过程中,代码使用一个 while 循环来找到当前元素应该插入的位置,并在找到位置后将其插入。最后,代码输出该元素插入的位置。
修改下列代码 public class ForEach1 { public static void main (String args[] ) { int sum = 0; int []nums = {1,2,3,4,5,6,7,8,9,0}; for(【代码】){ //依次遍历数组nums的元素 System.out.println(“数组元素:”+i); sum+=i; } System.out.println(“数组元素和:”+sum); } }
修改代码如下:
public class ForEach1 {
public static void main (String args[] ) {
int sum = 0;
int []nums = {1,2,3,4,5,6,7,8,9,0};
for(int i : nums){ //使用增强型for循环依次遍历数组nums的元素
System.out.println("数组元素:" + i);
sum += i; //累加数组元素到sum变量中
}
System.out.println("数组元素和:" + sum);
}
}
修改后的程序使用增强型for循环遍历数组元素,并累加元素到sum变量中,最后输出数组元素和sum。
阅读全文
相关推荐
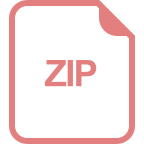
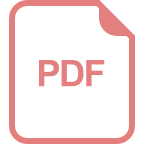
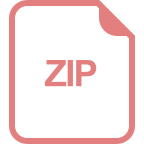













