能否提供一个用于猫狗图像分类的详细代码示例,包括使用的编程语言和相关的深度学习库?
时间: 2024-12-29 15:33:58 浏览: 10
当然可以。这里以Python语言和常用的深度学习库TensorFlow为例,给出一个简单的猫狗图像分类的Keras模型实现。假设我们已经准备好了训练集和测试集数据(如CIFAR-10数据集),并且数据预处理已完成。
```python
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D
from tensorflow.keras.models import Model
# 加载预训练的ResNet50模型,去掉顶部的全连接层
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=(150, 150, 3))
# 添加全局平均池化层和新全连接层
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(1024, activation='relu')(x) # 输出维度为1024,可以根据需要调整
predictions = Dense(2, activation='softmax') # 二分类,2个类别:猫和狗
final_model = Model(inputs=base_model.input, outputs=predictions)
# 冻结预训练模型的卷积层
for layer in base_model.layers:
layer.trainable = False
# 编译模型
final_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
# 这里省略了实际的数据加载和训练步骤,你可以使用tf.data API来加载并处理数据
# train_data = ... # 假设这是一个包含训练图片和标签的批次生成器
# final_model.fit(train_data, epochs=数 epochs, validation_data=val_data)
# 对新的图像进行预测
def predict_image(image_path):
image = tf.keras.preprocessing.image.load_img(image_path, target_size=(150, 150))
image_array = tf.keras.preprocessing.image.img_to_array(image)
image_array = np.expand_dims(image_array, axis=0)
image_array = preprocess_input(image_array)
prediction = final_model.predict(image_array)
class_index = np.argmax(prediction)
return "This is a {} with {:.2f}% confidence.".format("cat" if class_index == 0 else "dog", prediction[class_index] * 100)
# 使用predict_image函数对图像进行分类
new_image_path = 'path_to_your_cat_or_dog_image.jpg'
print(predict_image(new_image_path))
```
阅读全文
相关推荐



















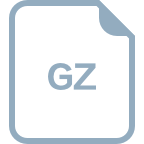