在C语言中如何详细实现单链表的创建、插入、查找与删除功能?请提供详细代码。
时间: 2024-11-23 16:43:46 浏览: 2
单链表作为一种基础的数据结构,在C语言中有着广泛的应用。为了帮助你更好地理解单链表的操作,推荐参考以下资源:《C语言实现单链表基本操作》。这份资料将为你提供具体的代码实现,以及每个操作背后的细节。
参考资源链接:[C语言实现单链表基本操作](https://wenku.csdn.net/doc/4swdhz1ujw?spm=1055.2569.3001.10343)
首先,创建一个单链表需要定义链表节点的结构体,以及链表的头指针。链表节点结构体通常包含数据域和指向下一个节点的指针。创建单链表的基本步骤如下:
```c
typedef struct Node {
char data; // 数据域
struct Node* next; // 指针域
} Node;
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node)); // 创建头节点
if (head == NULL) {
exit(EXIT_FAILURE);
}
head->next = NULL;
return head;
}
```
接下来,实现插入节点的操作。该操作通常涉及到定位到链表的特定位置,并创建新节点插入其中:
```c
void insertNode(Node* head, char data, int position) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
exit(EXIT_FAILURE);
}
newNode->data = data;
Node* current = head;
for (int i = 0; i < position && current != NULL; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
```
查找元素需要遍历链表直到找到目标数据或者链表结束:
```c
Node* findNode(Node* head, char data) {
Node* current = head->next; // 从头节点的下一个节点开始
while (current != NULL) {
if (current->data == data) {
return current;
}
current = current->next;
}
return NULL; // 未找到返回NULL
}
```
最后,删除节点需要先找到要删除节点的前一个节点,然后调整指针来排除目标节点,并释放其内存:
```c
void deleteNode(Node* head, int position) {
if (position < 0) return;
Node* current = head;
for (int i = 0; current != NULL && i < position; i++) {
current = current->next;
}
if (current == NULL || current->next == NULL) {
return; // 没有找到或已到链表末尾
}
Node* next = current->next->next;
free(current->next);
current->next = next;
}
```
上述代码展示了如何在C语言中实现单链表的创建、插入、查找和删除操作。每一个函数都涉及到对链表节点指针的操作,这是实现动态数据结构的核心。通过这些操作,可以构建出一个灵活且功能强大的单链表。如果你希望更深入地了解链表操作或者内存管理的细节,请查阅《C语言实现单链表基本操作》一书,它将为你提供更加全面的指导和解释。
参考资源链接:[C语言实现单链表基本操作](https://wenku.csdn.net/doc/4swdhz1ujw?spm=1055.2569.3001.10343)
阅读全文
相关推荐
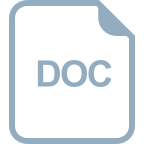
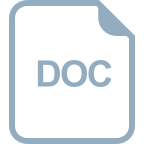
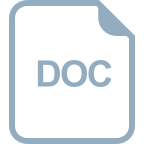
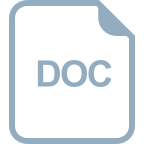
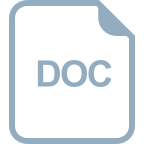
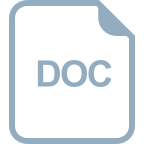
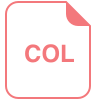
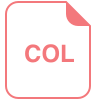










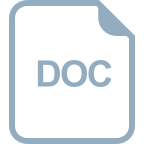