用c语言链表编译图书管理系统包含库存量、借阅量、入库时间、借阅时间、统计书籍借阅情、库存情况,且不包含文件
时间: 2023-07-10 18:14:59 浏览: 94
好的,我可以为您提供一个基本的图书管理系统的代码,包含库存量、借阅量、入库时间、借阅时间、统计书籍借阅情况和库存情况等功能。
我们依然使用链表来存储图书信息,只不过需要在结构体中添加新的字段。
这里我们定义一个包含库存量、借阅量、入库时间、借阅时间、统计书籍借阅情况和库存情况等字段的图书结构体,然后使用链表来存储这些图书信息。
下面是一个基本的代码框架:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
//定义图书结构体
typedef struct book {
char name[50];
char author[20];
float price;
int stock; //库存量
int borrow; //借阅量
char in_time[20]; //入库时间
char borrow_time[20]; //借阅时间
struct book *next;
} Book;
//链表头指针
Book *head = NULL;
//添加图书
void add_book() {
Book *p = (Book*)malloc(sizeof(Book));
printf("请输入书名:");
scanf("%s", p->name);
printf("请输入作者:");
scanf("%s", p->author);
printf("请输入价格:");
scanf("%f", &p->price);
printf("请输入库存量:");
scanf("%d", &p->stock);
printf("请输入入库时间:");
scanf("%s", p->in_time);
p->borrow = 0;
strcpy(p->borrow_time, "未借阅");
p->next = head;
head = p;
printf("添加成功!\n");
}
//删除图书
void delete_book() {
char name[50];
printf("请输入要删除的图书名称:");
scanf("%s", name);
Book *p = head;
Book *q = NULL;
while (p != NULL && strcmp(p->name, name) != 0) {
q = p;
p = p->next;
}
if (p == NULL) {
printf("未找到该图书!\n");
return;
}
if (q == NULL) {
head = p->next;
} else {
q->next = p->next;
}
free(p);
printf("删除成功!\n");
}
//查找图书
void find_book() {
char name[50];
printf("请输入要查找的图书名称:");
scanf("%s", name);
Book *p = head;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
}
if (p == NULL) {
printf("未找到该图书!\n");
return;
}
printf("书名:%s,作者:%s,价格:%.2f,库存量:%d,借阅量:%d,入库时间:%s,借阅时间:%s\n",
p->name, p->author, p->price, p->stock, p->borrow, p->in_time, p->borrow_time);
}
//借阅图书
void borrow_book() {
char name[50];
printf("请输入要借阅的图书名称:");
scanf("%s", name);
Book *p = head;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
}
if (p == NULL) {
printf("未找到该图书!\n");
return;
}
if (p->stock == 0) {
printf("该图书已借完!\n");
return;
}
p->stock--;
p->borrow++;
printf("借阅成功!\n");
}
//归还图书
void return_book() {
char name[50];
printf("请输入要归还的图书名称:");
scanf("%s", name);
Book *p = head;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
}
if (p == NULL) {
printf("未找到该图书!\n");
return;
}
if (p->borrow == 0) {
printf("该图书未被借阅!\n");
return;
}
p->stock++;
p->borrow--;
printf("归还成功!\n");
}
//统计借阅情况
void count_borrow() {
int total = 0;
Book *p = head;
while (p != NULL) {
total += p->borrow;
p = p->next;
}
printf("总借阅量为:%d\n", total);
}
//统计库存情况
void count_stock() {
int total = 0;
Book *p = head;
while (p != NULL) {
total += p->stock;
p = p->next;
}
printf("总库存量为:%d\n", total);
}
//显示所有图书
void show_books() {
if (head == NULL) {
printf("暂无图书!\n");
return;
}
Book *p = head;
while (p != NULL) {
printf("书名:%s,作者:%s,价格:%.2f,库存量:%d,借阅量:%d,入库时间:%s,借阅时间:%s\n",
p->name, p->author, p->price, p->stock, p->borrow, p->in_time, p->borrow_time);
p = p->next;
}
}
int main() {
int choice;
while (1) {
printf("请选择操作:\n");
printf("1.添加图书\n");
printf("2.删除图书\n");
printf("3.查找图书\n");
printf("4.借阅图书\n");
printf("5.归还图书\n");
printf("6.统计借阅情况\n");
printf("7.统计库存情况\n");
printf("8.显示所有图书\n");
printf("9.退出系统\n");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
delete_book();
break;
case 3:
find_book();
break;
case 4:
borrow_book();
break;
case 5:
return_book();
break;
case 6:
count_borrow();
break;
case 7:
count_stock();
break;
case 8:
show_books();
break;
case 9:
return 0;
default:
printf("输入有误,请重新输入!\n");
break;
}
}
return 0;
}
```
这是一个基本的图书管理系统,包含库存量、借阅量、入库时间、借阅时间、统计书籍借阅情况和库存情况等功能。你可以根据自己的需求来扩展功能,例如修改图书信息、排序等。同时,由于本程序没有使用文件来保存图书信息,所以程序结束后所有数据都会丢失,如果需要长期保存数据,可以使用文件来存储。
阅读全文
相关推荐
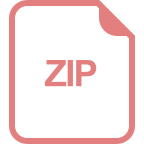
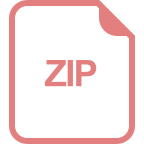


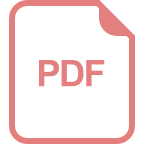
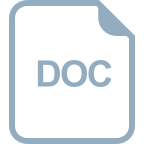
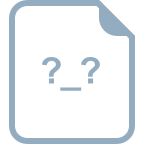
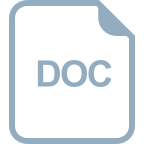
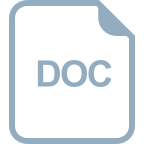
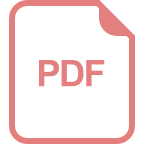
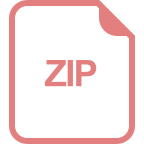
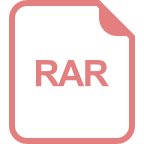
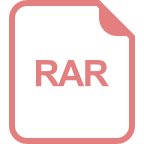
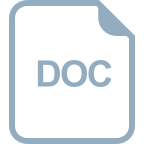
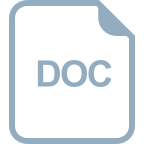
