<dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>3.9</version> </dependency> java代码创建多行表头 将第一行表头进行合并单元格
时间: 2023-06-17 20:06:11 浏览: 77
可以使用 Apache POI 库来创建多行表头并合并单元格。以下是示例代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.ss.util.CellRangeAddress;
public class CreateExcelFile {
public static void main(String[] args) throws IOException {
// 创建工作簿
Workbook workbook = new XSSFWorkbook();
// 创建工作表
Sheet sheet = workbook.createSheet("Test Sheet");
// 创建第一行表头
Row row1 = sheet.createRow(0);
CellStyle style = workbook.createCellStyle();
style.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
style.setFillPattern(CellStyle.SOLID_FOREGROUND);
// 合并第一行的前三列
CellRangeAddress mergedRegion = new CellRangeAddress(0, 0, 0, 2);
sheet.addMergedRegion(mergedRegion);
// 创建第一列的表头
Cell cell1 = row1.createCell(0);
cell1.setCellValue("Header 1");
cell1.setCellStyle(style);
// 创建第一行的第二列
Cell cell2 = row1.createCell(1);
cell2.setCellValue("Header 2");
cell2.setCellStyle(style);
// 创建第一行的第三列
Cell cell3 = row1.createCell(2);
cell3.setCellValue("Header 3");
cell3.setCellStyle(style);
// 创建第二行表头
Row row2 = sheet.createRow(1);
// 创建第二行的第一列
Cell cell4 = row2.createCell(0);
cell4.setCellValue("Subheader 1");
cell4.setCellStyle(style);
// 创建第二行的第二列
Cell cell5 = row2.createCell(1);
cell5.setCellValue("Subheader 2");
cell5.setCellStyle(style);
// 创建第二行的第三列
Cell cell6 = row2.createCell(2);
cell6.setCellValue("Subheader 3");
cell6.setCellStyle(style);
// 将工作簿写入文件
FileOutputStream outputStream = new FileOutputStream("test.xlsx");
workbook.write(outputStream);
workbook.close();
}
}
```
此代码将创建一个名为“Test Sheet”的工作表,并在第一行创建一个合并了前三列的表头。第一行的第一列将包含“Header 1”,第一行的第二列将包含“Header 2”,第一行的第三列将包含“Header 3”。第二行将包含第二层表头,第二行的第一列将包含“Subheader 1”,第二行的第二列将包含“Subheader 2”,第二行的第三列将包含“Subheader 3”。最后,工作簿将被写入名为“test.xlsx”的文件中。
阅读全文
相关推荐
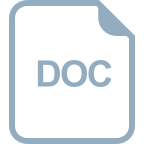
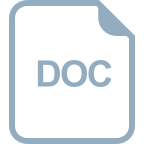
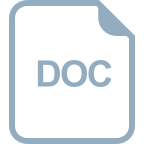






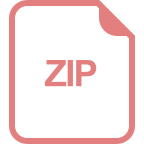
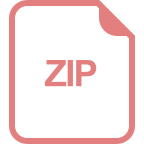
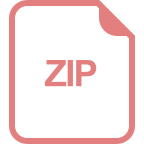
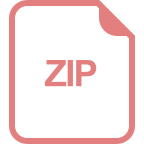
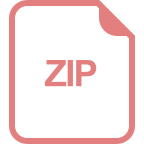
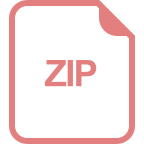
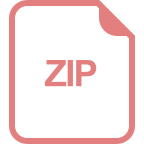
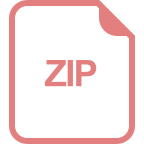
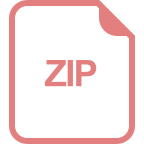
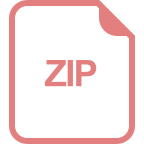